NOISSUE Add a skeleton of the wonko system
This commit is contained in:
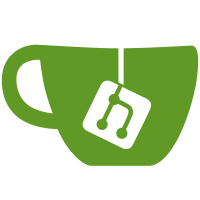
committed by
Petr Mrázek
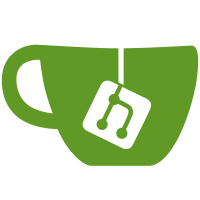
parent
5ae3b2c114
commit
00e5968bd2
@ -32,6 +32,7 @@ Config::Config()
|
||||
VERSION_STR = "@MultiMC_VERSION_STRING@";
|
||||
NEWS_RSS_URL = "@MultiMC_NEWS_RSS_URL@";
|
||||
PASTE_EE_KEY = "@MultiMC_PASTE_EE_API_KEY@";
|
||||
WONKO_ROOT_URL = "@MultiMC_WONKO_ROOT_URL@";
|
||||
}
|
||||
|
||||
QString Config::printableVersionString() const
|
||||
|
@ -57,6 +57,11 @@ public:
|
||||
*/
|
||||
QString PASTE_EE_KEY;
|
||||
|
||||
/**
|
||||
* Root URL for wonko things. Other wonko URLs will be resolved relative to this.
|
||||
*/
|
||||
QString WONKO_ROOT_URL;
|
||||
|
||||
/**
|
||||
* \brief Converts the Version to a string.
|
||||
* \return The version number in string format (major.minor.revision.build).
|
||||
|
@ -26,6 +26,10 @@ set(MultiMC_PASTE_EE_API_KEY "" CACHE STRING "API key you can get from paste.ee
|
||||
#### Check the current Git commit and branch
|
||||
include(GetGitRevisionDescription)
|
||||
get_git_head_revision(MultiMC_GIT_REFSPEC MultiMC_GIT_COMMIT)
|
||||
|
||||
# Root URL for wonko files
|
||||
set(MultiMC_WONKO_ROOT_URL "" CACHE STRING "Root URL for wonko stuff")
|
||||
|
||||
message(STATUS "Git commit: ${MultiMC_GIT_COMMIT}")
|
||||
message(STATUS "Git refspec: ${MultiMC_GIT_REFSPEC}")
|
||||
|
||||
@ -99,6 +103,8 @@ SET(MULTIMC_SOURCES
|
||||
VersionProxyModel.cpp
|
||||
ColorCache.h
|
||||
ColorCache.cpp
|
||||
WonkoGui.h
|
||||
WonkoGui.cpp
|
||||
|
||||
# GUI - windows
|
||||
MainWindow.h
|
||||
@ -163,6 +169,8 @@ SET(MULTIMC_SOURCES
|
||||
pages/global/ProxyPage.h
|
||||
pages/global/PasteEEPage.cpp
|
||||
pages/global/PasteEEPage.h
|
||||
pages/global/WonkoPage.cpp
|
||||
pages/global/WonkoPage.h
|
||||
|
||||
# GUI - dialogs
|
||||
dialogs/AboutDialog.cpp
|
||||
@ -256,6 +264,7 @@ SET(MULTIMC_UIS
|
||||
pages/global/MultiMCPage.ui
|
||||
pages/global/ProxyPage.ui
|
||||
pages/global/PasteEEPage.ui
|
||||
pages/global/WonkoPage.ui
|
||||
|
||||
# Dialogs
|
||||
dialogs/CopyInstanceDialog.ui
|
||||
|
@ -11,6 +11,8 @@ public:
|
||||
VersionFilterModel(VersionProxyModel *parent) : QSortFilterProxyModel(parent)
|
||||
{
|
||||
m_parent = parent;
|
||||
setSortRole(BaseVersionList::SortRole);
|
||||
sort(0, Qt::DescendingOrder);
|
||||
}
|
||||
|
||||
bool filterAcceptsRow(int source_row, const QModelIndex &source_parent) const
|
||||
@ -30,14 +32,11 @@ public:
|
||||
auto versionString = data.toString();
|
||||
if(it.value().exact)
|
||||
{
|
||||
if (versionString != it.value().string)
|
||||
{
|
||||
return false;
|
||||
}
|
||||
return versionString == it.value().string;
|
||||
}
|
||||
else if (!versionIsInInterval(versionString, it.value().string))
|
||||
else
|
||||
{
|
||||
return false;
|
||||
return versionIsInInterval(versionString, it.value().string);
|
||||
}
|
||||
}
|
||||
default:
|
||||
|
74
application/WonkoGui.cpp
Normal file
74
application/WonkoGui.cpp
Normal file
@ -0,0 +1,74 @@
|
||||
#include "WonkoGui.h"
|
||||
|
||||
#include "dialogs/ProgressDialog.h"
|
||||
#include "wonko/WonkoIndex.h"
|
||||
#include "wonko/WonkoVersionList.h"
|
||||
#include "wonko/WonkoVersion.h"
|
||||
#include "Env.h"
|
||||
|
||||
WonkoIndexPtr Wonko::ensureIndexLoaded(QWidget *parent)
|
||||
{
|
||||
if (!ENV.wonkoIndex()->isLocalLoaded())
|
||||
{
|
||||
ProgressDialog(parent).execWithTask(ENV.wonkoIndex()->localUpdateTask());
|
||||
if (!ENV.wonkoIndex()->isRemoteLoaded() && ENV.wonkoIndex()->lists().size() == 0)
|
||||
{
|
||||
ProgressDialog(parent).execWithTask(ENV.wonkoIndex()->remoteUpdateTask());
|
||||
}
|
||||
}
|
||||
return ENV.wonkoIndex();
|
||||
}
|
||||
|
||||
WonkoVersionListPtr Wonko::ensureVersionListExists(const QString &uid, QWidget *parent)
|
||||
{
|
||||
ensureIndexLoaded(parent);
|
||||
if (!ENV.wonkoIndex()->isRemoteLoaded() && !ENV.wonkoIndex()->hasUid(uid))
|
||||
{
|
||||
ProgressDialog(parent).execWithTask(ENV.wonkoIndex()->remoteUpdateTask());
|
||||
}
|
||||
return ENV.wonkoIndex()->getList(uid);
|
||||
}
|
||||
WonkoVersionListPtr Wonko::ensureVersionListLoaded(const QString &uid, QWidget *parent)
|
||||
{
|
||||
WonkoVersionListPtr list = ensureVersionListExists(uid, parent);
|
||||
if (!list)
|
||||
{
|
||||
return nullptr;
|
||||
}
|
||||
if (!list->isLocalLoaded())
|
||||
{
|
||||
ProgressDialog(parent).execWithTask(list->localUpdateTask());
|
||||
if (!list->isLocalLoaded())
|
||||
{
|
||||
ProgressDialog(parent).execWithTask(list->remoteUpdateTask());
|
||||
}
|
||||
}
|
||||
return list->isComplete() ? list : nullptr;
|
||||
}
|
||||
|
||||
WonkoVersionPtr Wonko::ensureVersionExists(const QString &uid, const QString &version, QWidget *parent)
|
||||
{
|
||||
WonkoVersionListPtr list = ensureVersionListLoaded(uid, parent);
|
||||
if (!list)
|
||||
{
|
||||
return nullptr;
|
||||
}
|
||||
return list->getVersion(version);
|
||||
}
|
||||
WonkoVersionPtr Wonko::ensureVersionLoaded(const QString &uid, const QString &version, QWidget *parent, const UpdateType update)
|
||||
{
|
||||
WonkoVersionPtr vptr = ensureVersionExists(uid, version, parent);
|
||||
if (!vptr)
|
||||
{
|
||||
return nullptr;
|
||||
}
|
||||
if (!vptr->isLocalLoaded() || update == AlwaysUpdate)
|
||||
{
|
||||
ProgressDialog(parent).execWithTask(vptr->localUpdateTask());
|
||||
if (!vptr->isLocalLoaded() || update == AlwaysUpdate)
|
||||
{
|
||||
ProgressDialog(parent).execWithTask(vptr->remoteUpdateTask());
|
||||
}
|
||||
}
|
||||
return vptr->isComplete() ? vptr : nullptr;
|
||||
}
|
28
application/WonkoGui.h
Normal file
28
application/WonkoGui.h
Normal file
@ -0,0 +1,28 @@
|
||||
#pragma once
|
||||
|
||||
#include <memory>
|
||||
|
||||
class QWidget;
|
||||
class QString;
|
||||
|
||||
using WonkoIndexPtr = std::shared_ptr<class WonkoIndex>;
|
||||
using WonkoVersionListPtr = std::shared_ptr<class WonkoVersionList>;
|
||||
using WonkoVersionPtr = std::shared_ptr<class WonkoVersion>;
|
||||
|
||||
namespace Wonko
|
||||
{
|
||||
enum UpdateType
|
||||
{
|
||||
AlwaysUpdate,
|
||||
UpdateIfNeeded
|
||||
};
|
||||
|
||||
/// Ensures that the index has been loaded, either from the local cache or remotely
|
||||
WonkoIndexPtr ensureIndexLoaded(QWidget *parent);
|
||||
/// Ensures that the given uid exists. Returns a nullptr if it doesn't.
|
||||
WonkoVersionListPtr ensureVersionListExists(const QString &uid, QWidget *parent);
|
||||
/// Ensures that the given uid exists and is loaded, either from the local cache or remotely. Returns nullptr if it doesn't exist or couldn't be loaded.
|
||||
WonkoVersionListPtr ensureVersionListLoaded(const QString &uid, QWidget *parent);
|
||||
WonkoVersionPtr ensureVersionExists(const QString &uid, const QString &version, QWidget *parent);
|
||||
WonkoVersionPtr ensureVersionLoaded(const QString &uid, const QString &version, QWidget *parent, const UpdateType update = UpdateIfNeeded);
|
||||
}
|
@ -97,6 +97,18 @@ int ProgressDialog::execWithTask(Task *task)
|
||||
}
|
||||
}
|
||||
|
||||
// TODO: only provide the unique_ptr overloads
|
||||
int ProgressDialog::execWithTask(std::unique_ptr<Task> &&task)
|
||||
{
|
||||
connect(this, &ProgressDialog::destroyed, task.get(), &Task::deleteLater);
|
||||
return execWithTask(task.release());
|
||||
}
|
||||
int ProgressDialog::execWithTask(std::unique_ptr<Task> &task)
|
||||
{
|
||||
connect(this, &ProgressDialog::destroyed, task.get(), &Task::deleteLater);
|
||||
return execWithTask(task.release());
|
||||
}
|
||||
|
||||
bool ProgressDialog::handleImmediateResult(QDialog::DialogCode &result)
|
||||
{
|
||||
if(task->isFinished())
|
||||
|
@ -16,6 +16,7 @@
|
||||
#pragma once
|
||||
|
||||
#include <QDialog>
|
||||
#include <memory>
|
||||
|
||||
class Task;
|
||||
|
||||
@ -35,6 +36,8 @@ public:
|
||||
void updateSize();
|
||||
|
||||
int execWithTask(Task *task);
|
||||
int execWithTask(std::unique_ptr<Task> &&task);
|
||||
int execWithTask(std::unique_ptr<Task> &task);
|
||||
|
||||
void setSkipButton(bool present, QString label = QString());
|
||||
|
||||
|
240
application/pages/global/WonkoPage.cpp
Normal file
240
application/pages/global/WonkoPage.cpp
Normal file
@ -0,0 +1,240 @@
|
||||
/* Copyright 2015 MultiMC Contributors
|
||||
*
|
||||
* Licensed under the Apache License, Version 2.0 (the "License");
|
||||
* you may not use this file except in compliance with the License.
|
||||
* You may obtain a copy of the License at
|
||||
*
|
||||
* http://www.apache.org/licenses/LICENSE-2.0
|
||||
*
|
||||
* Unless required by applicable law or agreed to in writing, software
|
||||
* distributed under the License is distributed on an "AS IS" BASIS,
|
||||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
||||
* See the License for the specific language governing permissions and
|
||||
* limitations under the License.
|
||||
*/
|
||||
|
||||
#include "WonkoPage.h"
|
||||
#include "ui_WonkoPage.h"
|
||||
|
||||
#include <QDateTime>
|
||||
#include <QSortFilterProxyModel>
|
||||
#include <QRegularExpression>
|
||||
|
||||
#include "dialogs/ProgressDialog.h"
|
||||
#include "VersionProxyModel.h"
|
||||
|
||||
#include "wonko/WonkoIndex.h"
|
||||
#include "wonko/WonkoVersionList.h"
|
||||
#include "wonko/WonkoVersion.h"
|
||||
#include "Env.h"
|
||||
#include "MultiMC.h"
|
||||
|
||||
static QString formatRequires(const WonkoVersionPtr &version)
|
||||
{
|
||||
QStringList lines;
|
||||
for (const WonkoReference &ref : version->requires())
|
||||
{
|
||||
const QString readable = ENV.wonkoIndex()->hasUid(ref.uid()) ? ENV.wonkoIndex()->getList(ref.uid())->humanReadable() : ref.uid();
|
||||
if (ref.version().isEmpty())
|
||||
{
|
||||
lines.append(readable);
|
||||
}
|
||||
else
|
||||
{
|
||||
lines.append(QString("%1 (%2)").arg(readable, ref.version()));
|
||||
}
|
||||
}
|
||||
return lines.join('\n');
|
||||
}
|
||||
|
||||
WonkoPage::WonkoPage(QWidget *parent) :
|
||||
QWidget(parent),
|
||||
ui(new Ui::WonkoPage)
|
||||
{
|
||||
ui->setupUi(this);
|
||||
ui->tabWidget->tabBar()->hide();
|
||||
|
||||
m_fileProxy = new QSortFilterProxyModel(this);
|
||||
m_fileProxy->setSortRole(Qt::DisplayRole);
|
||||
m_fileProxy->setSortCaseSensitivity(Qt::CaseInsensitive);
|
||||
m_fileProxy->setFilterCaseSensitivity(Qt::CaseInsensitive);
|
||||
m_fileProxy->setFilterRole(Qt::DisplayRole);
|
||||
m_fileProxy->setFilterKeyColumn(0);
|
||||
m_fileProxy->sort(0);
|
||||
m_fileProxy->setSourceModel(ENV.wonkoIndex().get());
|
||||
ui->indexView->setModel(m_fileProxy);
|
||||
|
||||
m_filterProxy = new QSortFilterProxyModel(this);
|
||||
m_filterProxy->setSortRole(WonkoVersionList::SortRole);
|
||||
m_filterProxy->setFilterCaseSensitivity(Qt::CaseInsensitive);
|
||||
m_filterProxy->setFilterRole(Qt::DisplayRole);
|
||||
m_filterProxy->setFilterKeyColumn(0);
|
||||
m_filterProxy->sort(0, Qt::DescendingOrder);
|
||||
ui->versionsView->setModel(m_filterProxy);
|
||||
|
||||
m_versionProxy = new VersionProxyModel(this);
|
||||
m_filterProxy->setSourceModel(m_versionProxy);
|
||||
|
||||
connect(ui->indexView->selectionModel(), &QItemSelectionModel::currentChanged, this, &WonkoPage::updateCurrentVersionList);
|
||||
connect(ui->versionsView->selectionModel(), &QItemSelectionModel::currentChanged, this, &WonkoPage::updateVersion);
|
||||
connect(m_filterProxy, &QSortFilterProxyModel::dataChanged, this, &WonkoPage::versionListDataChanged);
|
||||
|
||||
updateCurrentVersionList(QModelIndex());
|
||||
updateVersion();
|
||||
}
|
||||
|
||||
WonkoPage::~WonkoPage()
|
||||
{
|
||||
delete ui;
|
||||
}
|
||||
|
||||
QIcon WonkoPage::icon() const
|
||||
{
|
||||
return MMC->getThemedIcon("looney");
|
||||
}
|
||||
|
||||
void WonkoPage::on_refreshIndexBtn_clicked()
|
||||
{
|
||||
ProgressDialog(this).execWithTask(ENV.wonkoIndex()->remoteUpdateTask());
|
||||
}
|
||||
void WonkoPage::on_refreshFileBtn_clicked()
|
||||
{
|
||||
WonkoVersionListPtr list = ui->indexView->currentIndex().data(WonkoIndex::ListPtrRole).value<WonkoVersionListPtr>();
|
||||
if (!list)
|
||||
{
|
||||
return;
|
||||
}
|
||||
ProgressDialog(this).execWithTask(list->remoteUpdateTask());
|
||||
}
|
||||
void WonkoPage::on_refreshVersionBtn_clicked()
|
||||
{
|
||||
WonkoVersionPtr version = ui->versionsView->currentIndex().data(WonkoVersionList::WonkoVersionPtrRole).value<WonkoVersionPtr>();
|
||||
if (!version)
|
||||
{
|
||||
return;
|
||||
}
|
||||
ProgressDialog(this).execWithTask(version->remoteUpdateTask());
|
||||
}
|
||||
|
||||
void WonkoPage::on_fileSearchEdit_textChanged(const QString &search)
|
||||
{
|
||||
if (search.isEmpty())
|
||||
{
|
||||
m_fileProxy->setFilterFixedString(QString());
|
||||
}
|
||||
else
|
||||
{
|
||||
QStringList parts = search.split(' ');
|
||||
std::transform(parts.begin(), parts.end(), parts.begin(), &QRegularExpression::escape);
|
||||
m_fileProxy->setFilterRegExp(".*" + parts.join(".*") + ".*");
|
||||
}
|
||||
}
|
||||
void WonkoPage::on_versionSearchEdit_textChanged(const QString &search)
|
||||
{
|
||||
if (search.isEmpty())
|
||||
{
|
||||
m_filterProxy->setFilterFixedString(QString());
|
||||
}
|
||||
else
|
||||
{
|
||||
QStringList parts = search.split(' ');
|
||||
std::transform(parts.begin(), parts.end(), parts.begin(), &QRegularExpression::escape);
|
||||
m_filterProxy->setFilterRegExp(".*" + parts.join(".*") + ".*");
|
||||
}
|
||||
}
|
||||
|
||||
void WonkoPage::updateCurrentVersionList(const QModelIndex &index)
|
||||
{
|
||||
if (index.isValid())
|
||||
{
|
||||
WonkoVersionListPtr list = index.data(WonkoIndex::ListPtrRole).value<WonkoVersionListPtr>();
|
||||
ui->versionsBox->setEnabled(true);
|
||||
ui->refreshFileBtn->setEnabled(true);
|
||||
ui->fileUidLabel->setEnabled(true);
|
||||
ui->fileUid->setText(list->uid());
|
||||
ui->fileNameLabel->setEnabled(true);
|
||||
ui->fileName->setText(list->name());
|
||||
m_versionProxy->setSourceModel(list.get());
|
||||
ui->refreshFileBtn->setText(tr("Refresh %1").arg(list->humanReadable()));
|
||||
|
||||
if (!list->isLocalLoaded())
|
||||
{
|
||||
std::unique_ptr<Task> task = list->localUpdateTask();
|
||||
connect(task.get(), &Task::finished, this, [this, list]()
|
||||
{
|
||||
if (list->count() == 0 && !list->isRemoteLoaded())
|
||||
{
|
||||
ProgressDialog(this).execWithTask(list->remoteUpdateTask());
|
||||
}
|
||||
});
|
||||
ProgressDialog(this).execWithTask(task);
|
||||
}
|
||||
}
|
||||
else
|
||||
{
|
||||
ui->versionsBox->setEnabled(false);
|
||||
ui->refreshFileBtn->setEnabled(false);
|
||||
ui->fileUidLabel->setEnabled(false);
|
||||
ui->fileUid->clear();
|
||||
ui->fileNameLabel->setEnabled(false);
|
||||
ui->fileName->clear();
|
||||
m_versionProxy->setSourceModel(nullptr);
|
||||
ui->refreshFileBtn->setText(tr("Refresh ___"));
|
||||
}
|
||||
}
|
||||
|
||||
void WonkoPage::versionListDataChanged(const QModelIndex &tl, const QModelIndex &br)
|
||||
{
|
||||
if (QItemSelection(tl, br).contains(ui->versionsView->currentIndex()))
|
||||
{
|
||||
updateVersion();
|
||||
}
|
||||
}
|
||||
|
||||
void WonkoPage::updateVersion()
|
||||
{
|
||||
WonkoVersionPtr version = std::dynamic_pointer_cast<WonkoVersion>(
|
||||
ui->versionsView->currentIndex().data(WonkoVersionList::VersionPointerRole).value<BaseVersionPtr>());
|
||||
if (version)
|
||||
{
|
||||
ui->refreshVersionBtn->setEnabled(true);
|
||||
ui->versionVersionLabel->setEnabled(true);
|
||||
ui->versionVersion->setText(version->version());
|
||||
ui->versionTimeLabel->setEnabled(true);
|
||||
ui->versionTime->setText(version->time().toString("yyyy-MM-dd HH:mm"));
|
||||
ui->versionTypeLabel->setEnabled(true);
|
||||
ui->versionType->setText(version->type());
|
||||
ui->versionRequiresLabel->setEnabled(true);
|
||||
ui->versionRequires->setText(formatRequires(version));
|
||||
ui->refreshVersionBtn->setText(tr("Refresh %1").arg(version->version()));
|
||||
}
|
||||
else
|
||||
{
|
||||
ui->refreshVersionBtn->setEnabled(false);
|
||||
ui->versionVersionLabel->setEnabled(false);
|
||||
ui->versionVersion->clear();
|
||||
ui->versionTimeLabel->setEnabled(false);
|
||||
ui->versionTime->clear();
|
||||
ui->versionTypeLabel->setEnabled(false);
|
||||
ui->versionType->clear();
|
||||
ui->versionRequiresLabel->setEnabled(false);
|
||||
ui->versionRequires->clear();
|
||||
ui->refreshVersionBtn->setText(tr("Refresh ___"));
|
||||
}
|
||||
}
|
||||
|
||||
void WonkoPage::opened()
|
||||
{
|
||||
if (!ENV.wonkoIndex()->isLocalLoaded())
|
||||
{
|
||||
std::unique_ptr<Task> task = ENV.wonkoIndex()->localUpdateTask();
|
||||
connect(task.get(), &Task::finished, this, [this]()
|
||||
{
|
||||
if (!ENV.wonkoIndex()->isRemoteLoaded())
|
||||
{
|
||||
ProgressDialog(this).execWithTask(ENV.wonkoIndex()->remoteUpdateTask());
|
||||
}
|
||||
});
|
||||
ProgressDialog(this).execWithTask(task);
|
||||
}
|
||||
}
|
57
application/pages/global/WonkoPage.h
Normal file
57
application/pages/global/WonkoPage.h
Normal file
@ -0,0 +1,57 @@
|
||||
/* Copyright 2015 MultiMC Contributors
|
||||
*
|
||||
* Licensed under the Apache License, Version 2.0 (the "License");
|
||||
* you may not use this file except in compliance with the License.
|
||||
* You may obtain a copy of the License at
|
||||
*
|
||||
* http://www.apache.org/licenses/LICENSE-2.0
|
||||
*
|
||||
* Unless required by applicable law or agreed to in writing, software
|
||||
* distributed under the License is distributed on an "AS IS" BASIS,
|
||||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
||||
* See the License for the specific language governing permissions and
|
||||
* limitations under the License.
|
||||
*/
|
||||
|
||||
#pragma once
|
||||
|
||||
#include <QWidget>
|
||||
|
||||
#include "pages/BasePage.h"
|
||||
|
||||
namespace Ui {
|
||||
class WonkoPage;
|
||||
}
|
||||
|
||||
class QSortFilterProxyModel;
|
||||
class VersionProxyModel;
|
||||
|
||||
class WonkoPage : public QWidget, public BasePage
|
||||
{
|
||||
Q_OBJECT
|
||||
public:
|
||||
explicit WonkoPage(QWidget *parent = 0);
|
||||
~WonkoPage();
|
||||
|
||||
QString id() const override { return "wonko-global"; }
|
||||
QString displayName() const override { return tr("Wonko"); }
|
||||
QIcon icon() const override;
|
||||
void opened() override;
|
||||
|
||||
private slots:
|
||||
void on_refreshIndexBtn_clicked();
|
||||
void on_refreshFileBtn_clicked();
|
||||
void on_refreshVersionBtn_clicked();
|
||||
void on_fileSearchEdit_textChanged(const QString &search);
|
||||
void on_versionSearchEdit_textChanged(const QString &search);
|
||||
void updateCurrentVersionList(const QModelIndex &index);
|
||||
void versionListDataChanged(const QModelIndex &tl, const QModelIndex &br);
|
||||
|
||||
private:
|
||||
Ui::WonkoPage *ui;
|
||||
QSortFilterProxyModel *m_fileProxy;
|
||||
QSortFilterProxyModel *m_filterProxy;
|
||||
VersionProxyModel *m_versionProxy;
|
||||
|
||||
void updateVersion();
|
||||
};
|
252
application/pages/global/WonkoPage.ui
Normal file
252
application/pages/global/WonkoPage.ui
Normal file
@ -0,0 +1,252 @@
|
||||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<ui version="4.0">
|
||||
<class>WonkoPage</class>
|
||||
<widget class="QWidget" name="WonkoPage">
|
||||
<property name="geometry">
|
||||
<rect>
|
||||
<x>0</x>
|
||||
<y>0</y>
|
||||
<width>640</width>
|
||||
<height>480</height>
|
||||
</rect>
|
||||
</property>
|
||||
<property name="windowTitle">
|
||||
<string>Form</string>
|
||||
</property>
|
||||
<layout class="QHBoxLayout" name="horizontalLayout">
|
||||
<property name="leftMargin">
|
||||
<number>0</number>
|
||||
</property>
|
||||
<property name="topMargin">
|
||||
<number>0</number>
|
||||
</property>
|
||||
<property name="rightMargin">
|
||||
<number>0</number>
|
||||
</property>
|
||||
<property name="bottomMargin">
|
||||
<number>0</number>
|
||||
</property>
|
||||
<item>
|
||||
<widget class="QTabWidget" name="tabWidget">
|
||||
<property name="currentIndex">
|
||||
<number>0</number>
|
||||
</property>
|
||||
<widget class="QWidget" name="tab">
|
||||
<attribute name="title">
|
||||
<string>Tab 1</string>
|
||||
</attribute>
|
||||
<layout class="QGridLayout" name="gridLayout">
|
||||
<item row="1" column="2">
|
||||
<widget class="QGroupBox" name="versionsBox">
|
||||
<property name="title">
|
||||
<string>Versions</string>
|
||||
</property>
|
||||
<layout class="QVBoxLayout" name="verticalLayout_2">
|
||||
<item>
|
||||
<widget class="QLineEdit" name="versionSearchEdit">
|
||||
<property name="placeholderText">
|
||||
<string>Search...</string>
|
||||
</property>
|
||||
<property name="clearButtonEnabled">
|
||||
<bool>true</bool>
|
||||
</property>
|
||||
</widget>
|
||||
</item>
|
||||
<item>
|
||||
<widget class="QTreeView" name="versionsView">
|
||||
<property name="alternatingRowColors">
|
||||
<bool>true</bool>
|
||||
</property>
|
||||
<attribute name="headerVisible">
|
||||
<bool>false</bool>
|
||||
</attribute>
|
||||
</widget>
|
||||
</item>
|
||||
<item>
|
||||
<layout class="QHBoxLayout" name="horizontalLayout_4">
|
||||
<item>
|
||||
<widget class="QPushButton" name="refreshVersionBtn">
|
||||
<property name="text">
|
||||
<string>Refresh ___</string>
|
||||
</property>
|
||||
</widget>
|
||||
</item>
|
||||
</layout>
|
||||
</item>
|
||||
<item>
|
||||
<layout class="QFormLayout" name="formLayout_2">
|
||||
<item row="0" column="0">
|
||||
<widget class="QLabel" name="versionVersionLabel">
|
||||
<property name="text">
|
||||
<string>Version:</string>
|
||||
</property>
|
||||
</widget>
|
||||
</item>
|
||||
<item row="0" column="1">
|
||||
<widget class="QLabel" name="versionVersion">
|
||||
<property name="text">
|
||||
<string/>
|
||||
</property>
|
||||
</widget>
|
||||
</item>
|
||||
<item row="1" column="0">
|
||||
<widget class="QLabel" name="versionTimeLabel">
|
||||
<property name="text">
|
||||
<string>Time:</string>
|
||||
</property>
|
||||
</widget>
|
||||
</item>
|
||||
<item row="1" column="1">
|
||||
<widget class="QLabel" name="versionTime">
|
||||
<property name="text">
|
||||
<string/>
|
||||
</property>
|
||||
</widget>
|
||||
</item>
|
||||
<item row="2" column="0">
|
||||
<widget class="QLabel" name="versionTypeLabel">
|
||||
<property name="text">
|
||||
<string>Type:</string>
|
||||
</property>
|
||||
</widget>
|
||||
</item>
|
||||
<item row="2" column="1">
|
||||
<widget class="QLabel" name="versionType">
|
||||
<property name="text">
|
||||
<string/>
|
||||
</property>
|
||||
</widget>
|
||||
</item>
|
||||
<item row="3" column="0">
|
||||
<widget class="QLabel" name="versionRequiresLabel">
|
||||
<property name="text">
|
||||
<string>Dependencies:</string>
|
||||
</property>
|
||||
</widget>
|
||||
</item>
|
||||
<item row="3" column="1">
|
||||
<widget class="QLabel" name="versionRequires">
|
||||
<property name="text">
|
||||
<string/>
|
||||
</property>
|
||||
</widget>
|
||||
</item>
|
||||
</layout>
|
||||
</item>
|
||||
<item>
|
||||
<spacer name="verticalSpacer_2">
|
||||
<property name="orientation">
|
||||
<enum>Qt::Vertical</enum>
|
||||
</property>
|
||||
<property name="sizeHint" stdset="0">
|
||||
<size>
|
||||
<width>20</width>
|
||||
<height>40</height>
|
||||
</size>
|
||||
</property>
|
||||
</spacer>
|
||||
</item>
|
||||
</layout>
|
||||
</widget>
|
||||
</item>
|
||||
<item row="1" column="1">
|
||||
<widget class="QGroupBox" name="versionListsBox">
|
||||
<property name="title">
|
||||
<string>Resources</string>
|
||||
</property>
|
||||
<layout class="QVBoxLayout" name="verticalLayout">
|
||||
<item>
|
||||
<widget class="QLineEdit" name="fileSearchEdit">
|
||||
<property name="placeholderText">
|
||||
<string>Search...</string>
|
||||
</property>
|
||||
<property name="clearButtonEnabled">
|
||||
<bool>true</bool>
|
||||
</property>
|
||||
</widget>
|
||||
</item>
|
||||
<item>
|
||||
<widget class="QTreeView" name="indexView">
|
||||
<property name="alternatingRowColors">
|
||||
<bool>true</bool>
|
||||
</property>
|
||||
<attribute name="headerVisible">
|
||||
<bool>false</bool>
|
||||
</attribute>
|
||||
</widget>
|
||||
</item>
|
||||
<item>
|
||||
<layout class="QHBoxLayout" name="horizontalLayout_3">
|
||||
<item>
|
||||
<widget class="QPushButton" name="refreshFileBtn">
|
||||
<property name="text">
|
||||
<string>Refresh ___</string>
|
||||
</property>
|
||||
</widget>
|
||||
</item>
|
||||
</layout>
|
||||
</item>
|
||||
<item>
|
||||
<layout class="QFormLayout" name="formLayout">
|
||||
<item row="0" column="0">
|
||||
<widget class="QLabel" name="fileUidLabel">
|
||||
<property name="text">
|
||||
<string>UID:</string>
|
||||
</property>
|
||||
</widget>
|
||||
</item>
|
||||
<item row="0" column="1">
|
||||
<widget class="QLabel" name="fileUid">
|
||||
<property name="text">
|
||||
<string/>
|
||||
</property>
|
||||
</widget>
|
||||
</item>
|
||||
<item row="1" column="0">
|
||||
<widget class="QLabel" name="fileNameLabel">
|
||||
<property name="text">
|
||||
<string>Name:</string>
|
||||
</property>
|
||||
</widget>
|
||||
</item>
|
||||
<item row="1" column="1">
|
||||
<widget class="QLabel" name="fileName">
|
||||
<property name="text">
|
||||
<string/>
|
||||
</property>
|
||||
</widget>
|
||||
</item>
|
||||
</layout>
|
||||
</item>
|
||||
<item>
|
||||
<spacer name="verticalSpacer">
|
||||
<property name="orientation">
|
||||
<enum>Qt::Vertical</enum>
|
||||
</property>
|
||||
<property name="sizeHint" stdset="0">
|
||||
<size>
|
||||
<width>20</width>
|
||||
<height>40</height>
|
||||
</size>
|
||||
</property>
|
||||
</spacer>
|
||||
</item>
|
||||
</layout>
|
||||
</widget>
|
||||
</item>
|
||||
<item row="0" column="1" colspan="2">
|
||||
<widget class="QPushButton" name="refreshIndexBtn">
|
||||
<property name="text">
|
||||
<string>Refresh Index</string>
|
||||
</property>
|
||||
</widget>
|
||||
</item>
|
||||
</layout>
|
||||
</widget>
|
||||
</widget>
|
||||
</item>
|
||||
</layout>
|
||||
</widget>
|
||||
<resources/>
|
||||
<connections/>
|
||||
</ui>
|
BIN
application/resources/multimc/16x16/looney.png
Normal file
BIN
application/resources/multimc/16x16/looney.png
Normal file
Binary file not shown.
After Width: | Height: | Size: 802 B |
BIN
application/resources/multimc/256x256/looney.png
Normal file
BIN
application/resources/multimc/256x256/looney.png
Normal file
Binary file not shown.
After Width: | Height: | Size: 67 KiB |
BIN
application/resources/multimc/32x32/looney.png
Normal file
BIN
application/resources/multimc/32x32/looney.png
Normal file
Binary file not shown.
After Width: | Height: | Size: 2.1 KiB |
BIN
application/resources/multimc/64x64/looney.png
Normal file
BIN
application/resources/multimc/64x64/looney.png
Normal file
Binary file not shown.
After Width: | Height: | Size: 6.7 KiB |
@ -236,5 +236,11 @@
|
||||
|
||||
<!-- discord logo icon thing. from discord. traced from bitmap -->
|
||||
<file>scalable/discord.svg</file>
|
||||
|
||||
<!-- Looney, used for Wonko, Monty Python -->
|
||||
<file>16x16/looney.png</file>
|
||||
<file>32x32/looney.png</file>
|
||||
<file>64x64/looney.png</file>
|
||||
<file>256x256/looney.png</file>
|
||||
</qresource>
|
||||
</RCC>
|
||||
|
@ -72,7 +72,7 @@ QVariant BaseVersionList::data(const QModelIndex &index, int role) const
|
||||
}
|
||||
}
|
||||
|
||||
BaseVersionList::RoleList BaseVersionList::providesRoles()
|
||||
BaseVersionList::RoleList BaseVersionList::providesRoles() const
|
||||
{
|
||||
return {VersionPointerRole, VersionRole, VersionIdRole, TypeRole};
|
||||
}
|
||||
@ -87,3 +87,18 @@ int BaseVersionList::columnCount(const QModelIndex &parent) const
|
||||
{
|
||||
return 1;
|
||||
}
|
||||
|
||||
QHash<int, QByteArray> BaseVersionList::roleNames() const
|
||||
{
|
||||
QHash<int, QByteArray> roles = QAbstractListModel::roleNames();
|
||||
roles.insert(VersionRole, "version");
|
||||
roles.insert(VersionIdRole, "versionId");
|
||||
roles.insert(ParentGameVersionRole, "parentGameVersion");
|
||||
roles.insert(RecommendedRole, "recommended");
|
||||
roles.insert(LatestRole, "latest");
|
||||
roles.insert(TypeRole, "type");
|
||||
roles.insert(BranchRole, "branch");
|
||||
roles.insert(PathRole, "path");
|
||||
roles.insert(ArchitectureRole, "architecture");
|
||||
return roles;
|
||||
}
|
||||
|
@ -50,9 +50,10 @@ public:
|
||||
TypeRole,
|
||||
BranchRole,
|
||||
PathRole,
|
||||
ArchitectureRole
|
||||
ArchitectureRole,
|
||||
SortRole
|
||||
};
|
||||
typedef QList<ModelRoles> RoleList;
|
||||
typedef QList<int> RoleList;
|
||||
|
||||
explicit BaseVersionList(QObject *parent = 0);
|
||||
|
||||
@ -78,9 +79,10 @@ public:
|
||||
virtual QVariant data(const QModelIndex &index, int role) const;
|
||||
virtual int rowCount(const QModelIndex &parent) const;
|
||||
virtual int columnCount(const QModelIndex &parent) const;
|
||||
virtual QHash<int, QByteArray> roleNames() const override;
|
||||
|
||||
//! which roles are provided by this version list?
|
||||
virtual RoleList providesRoles();
|
||||
virtual RoleList providesRoles() const;
|
||||
|
||||
/*!
|
||||
* \brief Finds a version by its descriptor.
|
||||
|
@ -313,6 +313,27 @@ set(LOGIC_SOURCES
|
||||
tools/MCEditTool.cpp
|
||||
tools/MCEditTool.h
|
||||
|
||||
# Wonko
|
||||
wonko/tasks/BaseWonkoEntityRemoteLoadTask.cpp
|
||||
wonko/tasks/BaseWonkoEntityRemoteLoadTask.h
|
||||
wonko/tasks/BaseWonkoEntityLocalLoadTask.cpp
|
||||
wonko/tasks/BaseWonkoEntityLocalLoadTask.h
|
||||
wonko/format/WonkoFormatV1.cpp
|
||||
wonko/format/WonkoFormatV1.h
|
||||
wonko/format/WonkoFormat.cpp
|
||||
wonko/format/WonkoFormat.h
|
||||
wonko/BaseWonkoEntity.cpp
|
||||
wonko/BaseWonkoEntity.h
|
||||
wonko/WonkoVersionList.cpp
|
||||
wonko/WonkoVersionList.h
|
||||
wonko/WonkoVersion.cpp
|
||||
wonko/WonkoVersion.h
|
||||
wonko/WonkoIndex.cpp
|
||||
wonko/WonkoIndex.h
|
||||
wonko/WonkoUtil.cpp
|
||||
wonko/WonkoUtil.h
|
||||
wonko/WonkoReference.cpp
|
||||
wonko/WonkoReference.h
|
||||
)
|
||||
################################ COMPILE ################################
|
||||
|
||||
|
@ -8,6 +8,7 @@
|
||||
#include <QNetworkAccessManager>
|
||||
#include <QDebug>
|
||||
#include "tasks/Task.h"
|
||||
#include "wonko/WonkoIndex.h"
|
||||
#include <QDebug>
|
||||
|
||||
/*
|
||||
@ -138,6 +139,15 @@ void Env::registerVersionList(QString name, std::shared_ptr< BaseVersionList > v
|
||||
m_versionLists[name] = vlist;
|
||||
}
|
||||
|
||||
std::shared_ptr<WonkoIndex> Env::wonkoIndex()
|
||||
{
|
||||
if (!m_wonkoIndex)
|
||||
{
|
||||
m_wonkoIndex = std::make_shared<WonkoIndex>();
|
||||
}
|
||||
return m_wonkoIndex;
|
||||
}
|
||||
|
||||
|
||||
void Env::initHttpMetaCache()
|
||||
{
|
||||
@ -154,6 +164,7 @@ void Env::initHttpMetaCache()
|
||||
m_metacache->addBase("root", QDir::currentPath());
|
||||
m_metacache->addBase("translations", QDir("translations").absolutePath());
|
||||
m_metacache->addBase("icons", QDir("cache/icons").absolutePath());
|
||||
m_metacache->addBase("wonko", QDir("cache/wonko").absolutePath());
|
||||
m_metacache->Load();
|
||||
}
|
||||
|
||||
|
@ -11,6 +11,7 @@ class QNetworkAccessManager;
|
||||
class HttpMetaCache;
|
||||
class BaseVersionList;
|
||||
class BaseVersion;
|
||||
class WonkoIndex;
|
||||
|
||||
#if defined(ENV)
|
||||
#undef ENV
|
||||
@ -49,9 +50,17 @@ public:
|
||||
std::shared_ptr<BaseVersion> getVersion(QString component, QString version);
|
||||
|
||||
void registerVersionList(QString name, std::shared_ptr<BaseVersionList> vlist);
|
||||
|
||||
std::shared_ptr<WonkoIndex> wonkoIndex();
|
||||
|
||||
QString wonkoRootUrl() const { return m_wonkoRootUrl; }
|
||||
void setWonkoRootUrl(const QString &url) { m_wonkoRootUrl = url; }
|
||||
|
||||
protected:
|
||||
std::shared_ptr<QNetworkAccessManager> m_qnam;
|
||||
std::shared_ptr<HttpMetaCache> m_metacache;
|
||||
std::shared_ptr<IconList> m_icons;
|
||||
QMap<QString, std::shared_ptr<BaseVersionList>> m_versionLists;
|
||||
std::shared_ptr<WonkoIndex> m_wonkoIndex;
|
||||
QString m_wonkoRootUrl;
|
||||
};
|
||||
|
32
logic/Json.h
32
logic/Json.h
@ -113,9 +113,9 @@ template<> MULTIMC_LOGIC_EXPORT QUrl requireIsType<QUrl>(const QJsonValue &value
|
||||
// the following functions are higher level functions, that make use of the above functions for
|
||||
// type conversion
|
||||
template <typename T>
|
||||
T ensureIsType(const QJsonValue &value, const T default_, const QString &what = "Value")
|
||||
T ensureIsType(const QJsonValue &value, const T default_ = T(), const QString &what = "Value")
|
||||
{
|
||||
if (value.isUndefined())
|
||||
if (value.isUndefined() || value.isNull())
|
||||
{
|
||||
return default_;
|
||||
}
|
||||
@ -142,7 +142,7 @@ T requireIsType(const QJsonObject &parent, const QString &key, const QString &wh
|
||||
}
|
||||
|
||||
template <typename T>
|
||||
T ensureIsType(const QJsonObject &parent, const QString &key, const T default_, const QString &what = "__placeholder__")
|
||||
T ensureIsType(const QJsonObject &parent, const QString &key, const T default_ = T(), const QString &what = "__placeholder__")
|
||||
{
|
||||
const QString localWhat = QString(what).replace("__placeholder__", '\'' + key + '\'');
|
||||
if (!parent.contains(key))
|
||||
@ -153,10 +153,10 @@ T ensureIsType(const QJsonObject &parent, const QString &key, const T default_,
|
||||
}
|
||||
|
||||
template <typename T>
|
||||
QList<T> requireIsArrayOf(const QJsonDocument &doc)
|
||||
QVector<T> requireIsArrayOf(const QJsonDocument &doc)
|
||||
{
|
||||
const QJsonArray array = requireArray(doc);
|
||||
QList<T> out;
|
||||
QVector<T> out;
|
||||
for (const QJsonValue val : array)
|
||||
{
|
||||
out.append(requireIsType<T>(val, "Document"));
|
||||
@ -165,19 +165,19 @@ QList<T> requireIsArrayOf(const QJsonDocument &doc)
|
||||
}
|
||||
|
||||
template <typename T>
|
||||
QList<T> ensureIsArrayOf(const QJsonValue &value, const QString &what = "Value")
|
||||
QVector<T> ensureIsArrayOf(const QJsonValue &value, const QString &what = "Value")
|
||||
{
|
||||
const QJsonArray array = requireIsType<QJsonArray>(value, what);
|
||||
QList<T> out;
|
||||
const QJsonArray array = ensureIsType<QJsonArray>(value, QJsonArray(), what);
|
||||
QVector<T> out;
|
||||
for (const QJsonValue val : array)
|
||||
{
|
||||
out.append(ensureIsType<T>(val, what));
|
||||
out.append(requireIsType<T>(val, what));
|
||||
}
|
||||
return out;
|
||||
}
|
||||
|
||||
template <typename T>
|
||||
QList<T> ensureIsArrayOf(const QJsonValue &value, const QList<T> default_, const QString &what = "Value")
|
||||
QVector<T> ensureIsArrayOf(const QJsonValue &value, const QVector<T> default_, const QString &what = "Value")
|
||||
{
|
||||
if (value.isUndefined())
|
||||
{
|
||||
@ -188,19 +188,19 @@ QList<T> ensureIsArrayOf(const QJsonValue &value, const QList<T> default_, const
|
||||
|
||||
/// @throw JsonException
|
||||
template <typename T>
|
||||
QList<T> requireIsArrayOf(const QJsonObject &parent, const QString &key, const QString &what = "__placeholder__")
|
||||
QVector<T> requireIsArrayOf(const QJsonObject &parent, const QString &key, const QString &what = "__placeholder__")
|
||||
{
|
||||
const QString localWhat = QString(what).replace("__placeholder__", '\'' + key + '\'');
|
||||
if (!parent.contains(key))
|
||||
{
|
||||
throw JsonException(localWhat + "s parent does not contain " + localWhat);
|
||||
}
|
||||
return requireIsArrayOf<T>(parent.value(key), localWhat);
|
||||
return ensureIsArrayOf<T>(parent.value(key), localWhat);
|
||||
}
|
||||
|
||||
template <typename T>
|
||||
QList<T> ensureIsArrayOf(const QJsonObject &parent, const QString &key,
|
||||
const QList<T> &default_, const QString &what = "__placeholder__")
|
||||
QVector<T> ensureIsArrayOf(const QJsonObject &parent, const QString &key,
|
||||
const QVector<T> &default_ = QVector<T>(), const QString &what = "__placeholder__")
|
||||
{
|
||||
const QString localWhat = QString(what).replace("__placeholder__", '\'' + key + '\'');
|
||||
if (!parent.contains(key))
|
||||
@ -216,7 +216,7 @@ QList<T> ensureIsArrayOf(const QJsonObject &parent, const QString &key,
|
||||
{ \
|
||||
return requireIsType<TYPE>(value, what); \
|
||||
} \
|
||||
inline TYPE ensure##NAME(const QJsonValue &value, const TYPE default_, const QString &what = "Value") \
|
||||
inline TYPE ensure##NAME(const QJsonValue &value, const TYPE default_ = TYPE(), const QString &what = "Value") \
|
||||
{ \
|
||||
return ensureIsType<TYPE>(value, default_, what); \
|
||||
} \
|
||||
@ -224,7 +224,7 @@ QList<T> ensureIsArrayOf(const QJsonObject &parent, const QString &key,
|
||||
{ \
|
||||
return requireIsType<TYPE>(parent, key, what); \
|
||||
} \
|
||||
inline TYPE ensure##NAME(const QJsonObject &parent, const QString &key, const TYPE default_, const QString &what = "__placeholder") \
|
||||
inline TYPE ensure##NAME(const QJsonObject &parent, const QString &key, const TYPE default_ = TYPE(), const QString &what = "__placeholder") \
|
||||
{ \
|
||||
return ensureIsType<TYPE>(parent, key, default_, what); \
|
||||
}
|
||||
|
@ -77,7 +77,7 @@ QVariant JavaInstallList::data(const QModelIndex &index, int role) const
|
||||
}
|
||||
}
|
||||
|
||||
BaseVersionList::RoleList JavaInstallList::providesRoles()
|
||||
BaseVersionList::RoleList JavaInstallList::providesRoles() const
|
||||
{
|
||||
return {VersionPointerRole, VersionIdRole, VersionRole, RecommendedRole, PathRole, ArchitectureRole};
|
||||
}
|
||||
|
@ -41,7 +41,7 @@ public:
|
||||
virtual void sortVersions() override;
|
||||
|
||||
virtual QVariant data(const QModelIndex &index, int role) const override;
|
||||
virtual RoleList providesRoles() override;
|
||||
virtual RoleList providesRoles() const override;
|
||||
|
||||
public slots:
|
||||
virtual void updateListData(QList<BaseVersionPtr> versions) override;
|
||||
|
@ -307,7 +307,7 @@ QVariant MinecraftVersionList::data(const QModelIndex& index, int role) const
|
||||
}
|
||||
}
|
||||
|
||||
BaseVersionList::RoleList MinecraftVersionList::providesRoles()
|
||||
BaseVersionList::RoleList MinecraftVersionList::providesRoles() const
|
||||
{
|
||||
return {VersionPointerRole, VersionRole, VersionIdRole, RecommendedRole, LatestRole, TypeRole};
|
||||
}
|
||||
|
@ -52,7 +52,7 @@ public:
|
||||
virtual int count() const override;
|
||||
virtual void sortVersions() override;
|
||||
virtual QVariant data(const QModelIndex & index, int role) const override;
|
||||
virtual RoleList providesRoles() override;
|
||||
virtual RoleList providesRoles() const override;
|
||||
|
||||
virtual BaseVersionPtr getLatestStable() const override;
|
||||
virtual BaseVersionPtr getRecommended() const override;
|
||||
|
@ -89,7 +89,7 @@ QVariant ForgeVersionList::data(const QModelIndex &index, int role) const
|
||||
}
|
||||
}
|
||||
|
||||
QList<BaseVersionList::ModelRoles> ForgeVersionList::providesRoles()
|
||||
BaseVersionList::RoleList ForgeVersionList::providesRoles() const
|
||||
{
|
||||
return {VersionPointerRole, VersionRole, VersionIdRole, ParentGameVersionRole, RecommendedRole, BranchRole};
|
||||
}
|
||||
|
@ -47,7 +47,7 @@ public:
|
||||
ForgeVersionPtr findVersionByVersionNr(QString version);
|
||||
|
||||
virtual QVariant data(const QModelIndex &index, int role) const override;
|
||||
virtual QList<ModelRoles> providesRoles() override;
|
||||
virtual RoleList providesRoles() const override;
|
||||
|
||||
virtual int columnCount(const QModelIndex &parent) const override;
|
||||
|
||||
|
39
logic/wonko/BaseWonkoEntity.cpp
Normal file
39
logic/wonko/BaseWonkoEntity.cpp
Normal file
@ -0,0 +1,39 @@
|
||||
/* Copyright 2015 MultiMC Contributors
|
||||
*
|
||||
* Licensed under the Apache License, Version 2.0 (the "License");
|
||||
* you may not use this file except in compliance with the License.
|
||||
* You may obtain a copy of the License at
|
||||
*
|
||||
* http://www.apache.org/licenses/LICENSE-2.0
|
||||
*
|
||||
* Unless required by applicable law or agreed to in writing, software
|
||||
* distributed under the License is distributed on an "AS IS" BASIS,
|
||||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
||||
* See the License for the specific language governing permissions and
|
||||
* limitations under the License.
|
||||
*/
|
||||
|
||||
#include "BaseWonkoEntity.h"
|
||||
|
||||
#include "Json.h"
|
||||
#include "WonkoUtil.h"
|
||||
|
||||
BaseWonkoEntity::~BaseWonkoEntity()
|
||||
{
|
||||
}
|
||||
|
||||
void BaseWonkoEntity::store() const
|
||||
{
|
||||
Json::write(serialized(), Wonko::localWonkoDir().absoluteFilePath(localFilename()));
|
||||
}
|
||||
|
||||
void BaseWonkoEntity::notifyLocalLoadComplete()
|
||||
{
|
||||
m_localLoaded = true;
|
||||
store();
|
||||
}
|
||||
void BaseWonkoEntity::notifyRemoteLoadComplete()
|
||||
{
|
||||
m_remoteLoaded = true;
|
||||
store();
|
||||
}
|
51
logic/wonko/BaseWonkoEntity.h
Normal file
51
logic/wonko/BaseWonkoEntity.h
Normal file
@ -0,0 +1,51 @@
|
||||
/* Copyright 2015 MultiMC Contributors
|
||||
*
|
||||
* Licensed under the Apache License, Version 2.0 (the "License");
|
||||
* you may not use this file except in compliance with the License.
|
||||
* You may obtain a copy of the License at
|
||||
*
|
||||
* http://www.apache.org/licenses/LICENSE-2.0
|
||||
*
|
||||
* Unless required by applicable law or agreed to in writing, software
|
||||
* distributed under the License is distributed on an "AS IS" BASIS,
|
||||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
||||
* See the License for the specific language governing permissions and
|
||||
* limitations under the License.
|
||||
*/
|
||||
|
||||
#pragma once
|
||||
|
||||
#include <QObject>
|
||||
#include <memory>
|
||||
|
||||
#include "multimc_logic_export.h"
|
||||
|
||||
class Task;
|
||||
|
||||
class MULTIMC_LOGIC_EXPORT BaseWonkoEntity
|
||||
{
|
||||
public:
|
||||
virtual ~BaseWonkoEntity();
|
||||
|
||||
using Ptr = std::shared_ptr<BaseWonkoEntity>;
|
||||
|
||||
virtual std::unique_ptr<Task> remoteUpdateTask() = 0;
|
||||
virtual std::unique_ptr<Task> localUpdateTask() = 0;
|
||||
virtual void merge(const std::shared_ptr<BaseWonkoEntity> &other) = 0;
|
||||
|
||||
void store() const;
|
||||
virtual QString localFilename() const = 0;
|
||||
virtual QJsonObject serialized() const = 0;
|
||||
|
||||
bool isComplete() const { return m_localLoaded || m_remoteLoaded; }
|
||||
|
||||
bool isLocalLoaded() const { return m_localLoaded; }
|
||||
bool isRemoteLoaded() const { return m_remoteLoaded; }
|
||||
|
||||
void notifyLocalLoadComplete();
|
||||
void notifyRemoteLoadComplete();
|
||||
|
||||
private:
|
||||
bool m_localLoaded = false;
|
||||
bool m_remoteLoaded = false;
|
||||
};
|
147
logic/wonko/WonkoIndex.cpp
Normal file
147
logic/wonko/WonkoIndex.cpp
Normal file
@ -0,0 +1,147 @@
|
||||
/* Copyright 2015 MultiMC Contributors
|
||||
*
|
||||
* Licensed under the Apache License, Version 2.0 (the "License");
|
||||
* you may not use this file except in compliance with the License.
|
||||
* You may obtain a copy of the License at
|
||||
*
|
||||
* http://www.apache.org/licenses/LICENSE-2.0
|
||||
*
|
||||
* Unless required by applicable law or agreed to in writing, software
|
||||
* distributed under the License is distributed on an "AS IS" BASIS,
|
||||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
||||
* See the License for the specific language governing permissions and
|
||||
* limitations under the License.
|
||||
*/
|
||||
|
||||
#include "WonkoIndex.h"
|
||||
|
||||
#include "WonkoVersionList.h"
|
||||
#include "tasks/BaseWonkoEntityLocalLoadTask.h"
|
||||
#include "tasks/BaseWonkoEntityRemoteLoadTask.h"
|
||||
#include "format/WonkoFormat.h"
|
||||
|
||||
WonkoIndex::WonkoIndex(QObject *parent)
|
||||
: QAbstractListModel(parent)
|
||||
{
|
||||
}
|
||||
WonkoIndex::WonkoIndex(const QVector<WonkoVersionListPtr> &lists, QObject *parent)
|
||||
: QAbstractListModel(parent), m_lists(lists)
|
||||
{
|
||||
for (int i = 0; i < m_lists.size(); ++i)
|
||||
{
|
||||
m_uids.insert(m_lists.at(i)->uid(), m_lists.at(i));
|
||||
connectVersionList(i, m_lists.at(i));
|
||||
}
|
||||
}
|
||||
|
||||
QVariant WonkoIndex::data(const QModelIndex &index, int role) const
|
||||
{
|
||||
if (index.parent().isValid() || index.row() < 0 || index.row() >= m_lists.size())
|
||||
{
|
||||
return QVariant();
|
||||
}
|
||||
|
||||
WonkoVersionListPtr list = m_lists.at(index.row());
|
||||
switch (role)
|
||||
{
|
||||
case Qt::DisplayRole:
|
||||
switch (index.column())
|
||||
{
|
||||
case 0: return list->humanReadable();
|
||||
default: break;
|
||||
}
|
||||
case UidRole: return list->uid();
|
||||
case NameRole: return list->name();
|
||||
case ListPtrRole: return QVariant::fromValue(list);
|
||||
}
|
||||
return QVariant();
|
||||
}
|
||||
int WonkoIndex::rowCount(const QModelIndex &parent) const
|
||||
{
|
||||
return m_lists.size();
|
||||
}
|
||||
int WonkoIndex::columnCount(const QModelIndex &parent) const
|
||||
{
|
||||
return 1;
|
||||
}
|
||||
QVariant WonkoIndex::headerData(int section, Qt::Orientation orientation, int role) const
|
||||
{
|
||||
if (orientation == Qt::Horizontal && role == Qt::DisplayRole && section == 0)
|
||||
{
|
||||
return tr("Name");
|
||||
}
|
||||
else
|
||||
{
|
||||
return QVariant();
|
||||
}
|
||||
}
|
||||
|
||||
std::unique_ptr<Task> WonkoIndex::remoteUpdateTask()
|
||||
{
|
||||
return std::unique_ptr<WonkoIndexRemoteLoadTask>(new WonkoIndexRemoteLoadTask(this, this));
|
||||
}
|
||||
std::unique_ptr<Task> WonkoIndex::localUpdateTask()
|
||||
{
|
||||
return std::unique_ptr<WonkoIndexLocalLoadTask>(new WonkoIndexLocalLoadTask(this, this));
|
||||
}
|
||||
|
||||
QJsonObject WonkoIndex::serialized() const
|
||||
{
|
||||
return WonkoFormat::serializeIndex(this);
|
||||
}
|
||||
|
||||
bool WonkoIndex::hasUid(const QString &uid) const
|
||||
{
|
||||
return m_uids.contains(uid);
|
||||
}
|
||||
WonkoVersionListPtr WonkoIndex::getList(const QString &uid) const
|
||||
{
|
||||
return m_uids.value(uid, nullptr);
|
||||
}
|
||||
WonkoVersionListPtr WonkoIndex::getListGuaranteed(const QString &uid) const
|
||||
{
|
||||
return m_uids.value(uid, std::make_shared<WonkoVersionList>(uid));
|
||||
}
|
||||
|
||||
void WonkoIndex::merge(const Ptr &other)
|
||||
{
|
||||
const QVector<WonkoVersionListPtr> lists = std::dynamic_pointer_cast<WonkoIndex>(other)->m_lists;
|
||||
// initial load, no need to merge
|
||||
if (m_lists.isEmpty())
|
||||
{
|
||||
beginResetModel();
|
||||
m_lists = lists;
|
||||
for (int i = 0; i < lists.size(); ++i)
|
||||
{
|
||||
m_uids.insert(lists.at(i)->uid(), lists.at(i));
|
||||
connectVersionList(i, lists.at(i));
|
||||
}
|
||||
endResetModel();
|
||||
}
|
||||
else
|
||||
{
|
||||
for (const WonkoVersionListPtr &list : lists)
|
||||
{
|
||||
if (m_uids.contains(list->uid()))
|
||||
{
|
||||
m_uids[list->uid()]->merge(list);
|
||||
}
|
||||
else
|
||||
{
|
||||
beginInsertRows(QModelIndex(), m_lists.size(), m_lists.size());
|
||||
connectVersionList(m_lists.size(), list);
|
||||
m_lists.append(list);
|
||||
m_uids.insert(list->uid(), list);
|
||||
endInsertRows();
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
void WonkoIndex::connectVersionList(const int row, const WonkoVersionListPtr &list)
|
||||
{
|
||||
connect(list.get(), &WonkoVersionList::nameChanged, this, [this, row]()
|
||||
{
|
||||
emit dataChanged(index(row), index(row), QVector<int>() << Qt::DisplayRole);
|
||||
});
|
||||
}
|
68
logic/wonko/WonkoIndex.h
Normal file
68
logic/wonko/WonkoIndex.h
Normal file
@ -0,0 +1,68 @@
|
||||
/* Copyright 2015 MultiMC Contributors
|
||||
*
|
||||
* Licensed under the Apache License, Version 2.0 (the "License");
|
||||
* you may not use this file except in compliance with the License.
|
||||
* You may obtain a copy of the License at
|
||||
*
|
||||
* http://www.apache.org/licenses/LICENSE-2.0
|
||||
*
|
||||
* Unless required by applicable law or agreed to in writing, software
|
||||
* distributed under the License is distributed on an "AS IS" BASIS,
|
||||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
||||
* See the License for the specific language governing permissions and
|
||||
* limitations under the License.
|
||||
*/
|
||||
|
||||
#pragma once
|
||||
|
||||
#include <QAbstractListModel>
|
||||
#include <memory>
|
||||
|
||||
#include "BaseWonkoEntity.h"
|
||||
|
||||
#include "multimc_logic_export.h"
|
||||
|
||||
class Task;
|
||||
using WonkoVersionListPtr = std::shared_ptr<class WonkoVersionList>;
|
||||
|
||||
class MULTIMC_LOGIC_EXPORT WonkoIndex : public QAbstractListModel, public BaseWonkoEntity
|
||||
{
|
||||
Q_OBJECT
|
||||
public:
|
||||
explicit WonkoIndex(QObject *parent = nullptr);
|
||||
explicit WonkoIndex(const QVector<WonkoVersionListPtr> &lists, QObject *parent = nullptr);
|
||||
|
||||
enum
|
||||
{
|
||||
UidRole = Qt::UserRole,
|
||||
NameRole,
|
||||
ListPtrRole
|
||||
};
|
||||
|
||||
QVariant data(const QModelIndex &index, int role) const override;
|
||||
int rowCount(const QModelIndex &parent) const override;
|
||||
int columnCount(const QModelIndex &parent) const override;
|
||||
QVariant headerData(int section, Qt::Orientation orientation, int role) const override;
|
||||
|
||||
std::unique_ptr<Task> remoteUpdateTask() override;
|
||||
std::unique_ptr<Task> localUpdateTask() override;
|
||||
|
||||
QString localFilename() const override { return "index.json"; }
|
||||
QJsonObject serialized() const override;
|
||||
|
||||
// queries
|
||||
bool hasUid(const QString &uid) const;
|
||||
WonkoVersionListPtr getList(const QString &uid) const;
|
||||
WonkoVersionListPtr getListGuaranteed(const QString &uid) const;
|
||||
|
||||
QVector<WonkoVersionListPtr> lists() const { return m_lists; }
|
||||
|
||||
public: // for usage by parsers only
|
||||
void merge(const BaseWonkoEntity::Ptr &other);
|
||||
|
||||
private:
|
||||
QVector<WonkoVersionListPtr> m_lists;
|
||||
QHash<QString, WonkoVersionListPtr> m_uids;
|
||||
|
||||
void connectVersionList(const int row, const WonkoVersionListPtr &list);
|
||||
};
|
44
logic/wonko/WonkoReference.cpp
Normal file
44
logic/wonko/WonkoReference.cpp
Normal file
@ -0,0 +1,44 @@
|
||||
/* Copyright 2015 MultiMC Contributors
|
||||
*
|
||||
* Licensed under the Apache License, Version 2.0 (the "License");
|
||||
* you may not use this file except in compliance with the License.
|
||||
* You may obtain a copy of the License at
|
||||
*
|
||||
* http://www.apache.org/licenses/LICENSE-2.0
|
||||
*
|
||||
* Unless required by applicable law or agreed to in writing, software
|
||||
* distributed under the License is distributed on an "AS IS" BASIS,
|
||||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
||||
* See the License for the specific language governing permissions and
|
||||
* limitations under the License.
|
||||
*/
|
||||
|
||||
#include "WonkoReference.h"
|
||||
|
||||
WonkoReference::WonkoReference(const QString &uid)
|
||||
: m_uid(uid)
|
||||
{
|
||||
}
|
||||
|
||||
QString WonkoReference::uid() const
|
||||
{
|
||||
return m_uid;
|
||||
}
|
||||
|
||||
QString WonkoReference::version() const
|
||||
{
|
||||
return m_version;
|
||||
}
|
||||
void WonkoReference::setVersion(const QString &version)
|
||||
{
|
||||
m_version = version;
|
||||
}
|
||||
|
||||
bool WonkoReference::operator==(const WonkoReference &other) const
|
||||
{
|
||||
return m_uid == other.m_uid && m_version == other.m_version;
|
||||
}
|
||||
bool WonkoReference::operator!=(const WonkoReference &other) const
|
||||
{
|
||||
return m_uid != other.m_uid || m_version != other.m_version;
|
||||
}
|
41
logic/wonko/WonkoReference.h
Normal file
41
logic/wonko/WonkoReference.h
Normal file
@ -0,0 +1,41 @@
|
||||
/* Copyright 2015 MultiMC Contributors
|
||||
*
|
||||
* Licensed under the Apache License, Version 2.0 (the "License");
|
||||
* you may not use this file except in compliance with the License.
|
||||
* You may obtain a copy of the License at
|
||||
*
|
||||
* http://www.apache.org/licenses/LICENSE-2.0
|
||||
*
|
||||
* Unless required by applicable law or agreed to in writing, software
|
||||
* distributed under the License is distributed on an "AS IS" BASIS,
|
||||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
||||
* See the License for the specific language governing permissions and
|
||||
* limitations under the License.
|
||||
*/
|
||||
|
||||
#pragma once
|
||||
|
||||
#include <QString>
|
||||
#include <QMetaType>
|
||||
|
||||
#include "multimc_logic_export.h"
|
||||
|
||||
class MULTIMC_LOGIC_EXPORT WonkoReference
|
||||
{
|
||||
public:
|
||||
WonkoReference() {}
|
||||
explicit WonkoReference(const QString &uid);
|
||||
|
||||
QString uid() const;
|
||||
|
||||
QString version() const;
|
||||
void setVersion(const QString &version);
|
||||
|
||||
bool operator==(const WonkoReference &other) const;
|
||||
bool operator!=(const WonkoReference &other) const;
|
||||
|
||||
private:
|
||||
QString m_uid;
|
||||
QString m_version;
|
||||
};
|
||||
Q_DECLARE_METATYPE(WonkoReference)
|
47
logic/wonko/WonkoUtil.cpp
Normal file
47
logic/wonko/WonkoUtil.cpp
Normal file
@ -0,0 +1,47 @@
|
||||
/* Copyright 2015 MultiMC Contributors
|
||||
*
|
||||
* Licensed under the Apache License, Version 2.0 (the "License");
|
||||
* you may not use this file except in compliance with the License.
|
||||
* You may obtain a copy of the License at
|
||||
*
|
||||
* http://www.apache.org/licenses/LICENSE-2.0
|
||||
*
|
||||
* Unless required by applicable law or agreed to in writing, software
|
||||
* distributed under the License is distributed on an "AS IS" BASIS,
|
||||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
||||
* See the License for the specific language governing permissions and
|
||||
* limitations under the License.
|
||||
*/
|
||||
|
||||
#include "WonkoUtil.h"
|
||||
|
||||
#include <QUrl>
|
||||
#include <QDir>
|
||||
|
||||
#include "Env.h"
|
||||
|
||||
namespace Wonko
|
||||
{
|
||||
QUrl rootUrl()
|
||||
{
|
||||
return ENV.wonkoRootUrl();
|
||||
}
|
||||
QUrl indexUrl()
|
||||
{
|
||||
return rootUrl().resolved(QStringLiteral("index.json"));
|
||||
}
|
||||
QUrl versionListUrl(const QString &uid)
|
||||
{
|
||||
return rootUrl().resolved(uid + ".json");
|
||||
}
|
||||
QUrl versionUrl(const QString &uid, const QString &version)
|
||||
{
|
||||
return rootUrl().resolved(uid + "/" + version + ".json");
|
||||
}
|
||||
|
||||
QDir localWonkoDir()
|
||||
{
|
||||
return QDir("wonko");
|
||||
}
|
||||
|
||||
}
|
31
logic/wonko/WonkoUtil.h
Normal file
31
logic/wonko/WonkoUtil.h
Normal file
@ -0,0 +1,31 @@
|
||||
/* Copyright 2015 MultiMC Contributors
|
||||
*
|
||||
* Licensed under the Apache License, Version 2.0 (the "License");
|
||||
* you may not use this file except in compliance with the License.
|
||||
* You may obtain a copy of the License at
|
||||
*
|
||||
* http://www.apache.org/licenses/LICENSE-2.0
|
||||
*
|
||||
* Unless required by applicable law or agreed to in writing, software
|
||||
* distributed under the License is distributed on an "AS IS" BASIS,
|
||||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
||||
* See the License for the specific language governing permissions and
|
||||
* limitations under the License.
|
||||
*/
|
||||
|
||||
#pragma once
|
||||
|
||||
#include "multimc_logic_export.h"
|
||||
|
||||
class QUrl;
|
||||
class QString;
|
||||
class QDir;
|
||||
|
||||
namespace Wonko
|
||||
{
|
||||
MULTIMC_LOGIC_EXPORT QUrl rootUrl();
|
||||
MULTIMC_LOGIC_EXPORT QUrl indexUrl();
|
||||
MULTIMC_LOGIC_EXPORT QUrl versionListUrl(const QString &uid);
|
||||
MULTIMC_LOGIC_EXPORT QUrl versionUrl(const QString &uid, const QString &version);
|
||||
MULTIMC_LOGIC_EXPORT QDir localWonkoDir();
|
||||
}
|
102
logic/wonko/WonkoVersion.cpp
Normal file
102
logic/wonko/WonkoVersion.cpp
Normal file
@ -0,0 +1,102 @@
|
||||
/* Copyright 2015 MultiMC Contributors
|
||||
*
|
||||
* Licensed under the Apache License, Version 2.0 (the "License");
|
||||
* you may not use this file except in compliance with the License.
|
||||
* You may obtain a copy of the License at
|
||||
*
|
||||
* http://www.apache.org/licenses/LICENSE-2.0
|
||||
*
|
||||
* Unless required by applicable law or agreed to in writing, software
|
||||
* distributed under the License is distributed on an "AS IS" BASIS,
|
||||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
||||
* See the License for the specific language governing permissions and
|
||||
* limitations under the License.
|
||||
*/
|
||||
|
||||
#include "WonkoVersion.h"
|
||||
|
||||
#include <QDateTime>
|
||||
|
||||
#include "tasks/BaseWonkoEntityLocalLoadTask.h"
|
||||
#include "tasks/BaseWonkoEntityRemoteLoadTask.h"
|
||||
#include "format/WonkoFormat.h"
|
||||
|
||||
WonkoVersion::WonkoVersion(const QString &uid, const QString &version)
|
||||
: BaseVersion(), m_uid(uid), m_version(version)
|
||||
{
|
||||
}
|
||||
|
||||
QString WonkoVersion::descriptor()
|
||||
{
|
||||
return m_version;
|
||||
}
|
||||
QString WonkoVersion::name()
|
||||
{
|
||||
return m_version;
|
||||
}
|
||||
QString WonkoVersion::typeString() const
|
||||
{
|
||||
return m_type;
|
||||
}
|
||||
|
||||
QDateTime WonkoVersion::time() const
|
||||
{
|
||||
return QDateTime::fromMSecsSinceEpoch(m_time * 1000, Qt::UTC);
|
||||
}
|
||||
|
||||
std::unique_ptr<Task> WonkoVersion::remoteUpdateTask()
|
||||
{
|
||||
return std::unique_ptr<WonkoVersionRemoteLoadTask>(new WonkoVersionRemoteLoadTask(this, this));
|
||||
}
|
||||
std::unique_ptr<Task> WonkoVersion::localUpdateTask()
|
||||
{
|
||||
return std::unique_ptr<WonkoVersionLocalLoadTask>(new WonkoVersionLocalLoadTask(this, this));
|
||||
}
|
||||
|
||||
void WonkoVersion::merge(const std::shared_ptr<BaseWonkoEntity> &other)
|
||||
{
|
||||
WonkoVersionPtr version = std::dynamic_pointer_cast<WonkoVersion>(other);
|
||||
if (m_type != version->m_type)
|
||||
{
|
||||
setType(version->m_type);
|
||||
}
|
||||
if (m_time != version->m_time)
|
||||
{
|
||||
setTime(version->m_time);
|
||||
}
|
||||
if (m_requires != version->m_requires)
|
||||
{
|
||||
setRequires(version->m_requires);
|
||||
}
|
||||
|
||||
setData(version->m_data);
|
||||
}
|
||||
|
||||
QString WonkoVersion::localFilename() const
|
||||
{
|
||||
return m_uid + '/' + m_version + ".json";
|
||||
}
|
||||
QJsonObject WonkoVersion::serialized() const
|
||||
{
|
||||
return WonkoFormat::serializeVersion(this);
|
||||
}
|
||||
|
||||
void WonkoVersion::setType(const QString &type)
|
||||
{
|
||||
m_type = type;
|
||||
emit typeChanged();
|
||||
}
|
||||
void WonkoVersion::setTime(const qint64 time)
|
||||
{
|
||||
m_time = time;
|
||||
emit timeChanged();
|
||||
}
|
||||
void WonkoVersion::setRequires(const QVector<WonkoReference> &requires)
|
||||
{
|
||||
m_requires = requires;
|
||||
emit requiresChanged();
|
||||
}
|
||||
void WonkoVersion::setData(const VersionFilePtr &data)
|
||||
{
|
||||
m_data = data;
|
||||
}
|
83
logic/wonko/WonkoVersion.h
Normal file
83
logic/wonko/WonkoVersion.h
Normal file
@ -0,0 +1,83 @@
|
||||
/* Copyright 2015 MultiMC Contributors
|
||||
*
|
||||
* Licensed under the Apache License, Version 2.0 (the "License");
|
||||
* you may not use this file except in compliance with the License.
|
||||
* You may obtain a copy of the License at
|
||||
*
|
||||
* http://www.apache.org/licenses/LICENSE-2.0
|
||||
*
|
||||
* Unless required by applicable law or agreed to in writing, software
|
||||
* distributed under the License is distributed on an "AS IS" BASIS,
|
||||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
||||
* See the License for the specific language governing permissions and
|
||||
* limitations under the License.
|
||||
*/
|
||||
|
||||
#pragma once
|
||||
|
||||
#include "BaseVersion.h"
|
||||
#include "BaseWonkoEntity.h"
|
||||
|
||||
#include <QVector>
|
||||
#include <QStringList>
|
||||
#include <QJsonObject>
|
||||
#include <memory>
|
||||
|
||||
#include "minecraft/VersionFile.h"
|
||||
#include "WonkoReference.h"
|
||||
|
||||
#include "multimc_logic_export.h"
|
||||
|
||||
using WonkoVersionPtr = std::shared_ptr<class WonkoVersion>;
|
||||
|
||||
class MULTIMC_LOGIC_EXPORT WonkoVersion : public QObject, public BaseVersion, public BaseWonkoEntity
|
||||
{
|
||||
Q_OBJECT
|
||||
Q_PROPERTY(QString uid READ uid CONSTANT)
|
||||
Q_PROPERTY(QString version READ version CONSTANT)
|
||||
Q_PROPERTY(QString type READ type NOTIFY typeChanged)
|
||||
Q_PROPERTY(QDateTime time READ time NOTIFY timeChanged)
|
||||
Q_PROPERTY(QVector<WonkoReference> requires READ requires NOTIFY requiresChanged)
|
||||
public:
|
||||
explicit WonkoVersion(const QString &uid, const QString &version);
|
||||
|
||||
QString descriptor() override;
|
||||
QString name() override;
|
||||
QString typeString() const override;
|
||||
|
||||
QString uid() const { return m_uid; }
|
||||
QString version() const { return m_version; }
|
||||
QString type() const { return m_type; }
|
||||
QDateTime time() const;
|
||||
qint64 rawTime() const { return m_time; }
|
||||
QVector<WonkoReference> requires() const { return m_requires; }
|
||||
VersionFilePtr data() const { return m_data; }
|
||||
|
||||
std::unique_ptr<Task> remoteUpdateTask() override;
|
||||
std::unique_ptr<Task> localUpdateTask() override;
|
||||
void merge(const std::shared_ptr<BaseWonkoEntity> &other) override;
|
||||
|
||||
QString localFilename() const override;
|
||||
QJsonObject serialized() const override;
|
||||
|
||||
public: // for usage by format parsers only
|
||||
void setType(const QString &type);
|
||||
void setTime(const qint64 time);
|
||||
void setRequires(const QVector<WonkoReference> &requires);
|
||||
void setData(const VersionFilePtr &data);
|
||||
|
||||
signals:
|
||||
void typeChanged();
|
||||
void timeChanged();
|
||||
void requiresChanged();
|
||||
|
||||
private:
|
||||
QString m_uid;
|
||||
QString m_version;
|
||||
QString m_type;
|
||||
qint64 m_time;
|
||||
QVector<WonkoReference> m_requires;
|
||||
VersionFilePtr m_data;
|
||||
};
|
||||
|
||||
Q_DECLARE_METATYPE(WonkoVersionPtr)
|
283
logic/wonko/WonkoVersionList.cpp
Normal file
283
logic/wonko/WonkoVersionList.cpp
Normal file
@ -0,0 +1,283 @@
|
||||
/* Copyright 2015 MultiMC Contributors
|
||||
*
|
||||
* Licensed under the Apache License, Version 2.0 (the "License");
|
||||
* you may not use this file except in compliance with the License.
|
||||
* You may obtain a copy of the License at
|
||||
*
|
||||
* http://www.apache.org/licenses/LICENSE-2.0
|
||||
*
|
||||
* Unless required by applicable law or agreed to in writing, software
|
||||
* distributed under the License is distributed on an "AS IS" BASIS,
|
||||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
||||
* See the License for the specific language governing permissions and
|
||||
* limitations under the License.
|
||||
*/
|
||||
|
||||
#include "WonkoVersionList.h"
|
||||
|
||||
#include <QDateTime>
|
||||
|
||||
#include "WonkoVersion.h"
|
||||
#include "tasks/BaseWonkoEntityRemoteLoadTask.h"
|
||||
#include "tasks/BaseWonkoEntityLocalLoadTask.h"
|
||||
#include "format/WonkoFormat.h"
|
||||
#include "WonkoReference.h"
|
||||
|
||||
class WVLLoadTask : public Task
|
||||
{
|
||||
Q_OBJECT
|
||||
public:
|
||||
explicit WVLLoadTask(WonkoVersionList *list, QObject *parent = nullptr)
|
||||
: Task(parent), m_list(list)
|
||||
{
|
||||
}
|
||||
|
||||
bool canAbort() const override
|
||||
{
|
||||
return !m_currentTask || m_currentTask->canAbort();
|
||||
}
|
||||
bool abort() override
|
||||
{
|
||||
return m_currentTask->abort();
|
||||
}
|
||||
|
||||
private:
|
||||
void executeTask() override
|
||||
{
|
||||
if (!m_list->isLocalLoaded())
|
||||
{
|
||||
m_currentTask = m_list->localUpdateTask();
|
||||
connect(m_currentTask.get(), &Task::succeeded, this, &WVLLoadTask::next);
|
||||
}
|
||||
else
|
||||
{
|
||||
m_currentTask = m_list->remoteUpdateTask();
|
||||
connect(m_currentTask.get(), &Task::succeeded, this, &WVLLoadTask::emitSucceeded);
|
||||
}
|
||||
connect(m_currentTask.get(), &Task::status, this, &WVLLoadTask::setStatus);
|
||||
connect(m_currentTask.get(), &Task::progress, this, &WVLLoadTask::setProgress);
|
||||
connect(m_currentTask.get(), &Task::failed, this, &WVLLoadTask::emitFailed);
|
||||
m_currentTask->start();
|
||||
}
|
||||
|
||||
void next()
|
||||
{
|
||||
m_currentTask = m_list->remoteUpdateTask();
|
||||
connect(m_currentTask.get(), &Task::status, this, &WVLLoadTask::setStatus);
|
||||
connect(m_currentTask.get(), &Task::progress, this, &WVLLoadTask::setProgress);
|
||||
connect(m_currentTask.get(), &Task::succeeded, this, &WVLLoadTask::emitSucceeded);
|
||||
m_currentTask->start();
|
||||
}
|
||||
|
||||
WonkoVersionList *m_list;
|
||||
std::unique_ptr<Task> m_currentTask;
|
||||
};
|
||||
|
||||
WonkoVersionList::WonkoVersionList(const QString &uid, QObject *parent)
|
||||
: BaseVersionList(parent), m_uid(uid)
|
||||
{
|
||||
setObjectName("Wonko version list: " + uid);
|
||||
}
|
||||
|
||||
Task *WonkoVersionList::getLoadTask()
|
||||
{
|
||||
return new WVLLoadTask(this);
|
||||
}
|
||||
|
||||
bool WonkoVersionList::isLoaded()
|
||||
{
|
||||
return isLocalLoaded() && isRemoteLoaded();
|
||||
}
|
||||
|
||||
const BaseVersionPtr WonkoVersionList::at(int i) const
|
||||
{
|
||||
return m_versions.at(i);
|
||||
}
|
||||
int WonkoVersionList::count() const
|
||||
{
|
||||
return m_versions.size();
|
||||
}
|
||||
|
||||
void WonkoVersionList::sortVersions()
|
||||
{
|
||||
beginResetModel();
|
||||
std::sort(m_versions.begin(), m_versions.end(), [](const WonkoVersionPtr &a, const WonkoVersionPtr &b)
|
||||
{
|
||||
return *a.get() < *b.get();
|
||||
});
|
||||
endResetModel();
|
||||
}
|
||||
|
||||
QVariant WonkoVersionList::data(const QModelIndex &index, int role) const
|
||||
{
|
||||
if (!index.isValid() || index.row() < 0 || index.row() >= m_versions.size() || index.parent().isValid())
|
||||
{
|
||||
return QVariant();
|
||||
}
|
||||
|
||||
WonkoVersionPtr version = m_versions.at(index.row());
|
||||
|
||||
switch (role)
|
||||
{
|
||||
case VersionPointerRole: return QVariant::fromValue(std::dynamic_pointer_cast<BaseVersion>(version));
|
||||
case VersionRole:
|
||||
case VersionIdRole:
|
||||
return version->version();
|
||||
case ParentGameVersionRole:
|
||||
{
|
||||
const auto end = version->requires().end();
|
||||
const auto it = std::find_if(version->requires().begin(), end,
|
||||
[](const WonkoReference &ref) { return ref.uid() == "net.minecraft"; });
|
||||
if (it != end)
|
||||
{
|
||||
return (*it).version();
|
||||
}
|
||||
return QVariant();
|
||||
}
|
||||
case TypeRole: return version->type();
|
||||
|
||||
case UidRole: return version->uid();
|
||||
case TimeRole: return version->time();
|
||||
case RequiresRole: return QVariant::fromValue(version->requires());
|
||||
case SortRole: return version->rawTime();
|
||||
case WonkoVersionPtrRole: return QVariant::fromValue(version);
|
||||
case RecommendedRole: return version == getRecommended();
|
||||
case LatestRole: return version == getLatestStable();
|
||||
default: return QVariant();
|
||||
}
|
||||
}
|
||||
|
||||
BaseVersionList::RoleList WonkoVersionList::providesRoles() const
|
||||
{
|
||||
return {VersionPointerRole, VersionRole, VersionIdRole, ParentGameVersionRole,
|
||||
TypeRole, UidRole, TimeRole, RequiresRole, SortRole,
|
||||
RecommendedRole, LatestRole, WonkoVersionPtrRole};
|
||||
}
|
||||
|
||||
QHash<int, QByteArray> WonkoVersionList::roleNames() const
|
||||
{
|
||||
QHash<int, QByteArray> roles = BaseVersionList::roleNames();
|
||||
roles.insert(UidRole, "uid");
|
||||
roles.insert(TimeRole, "time");
|
||||
roles.insert(SortRole, "sort");
|
||||
roles.insert(RequiresRole, "requires");
|
||||
return roles;
|
||||
}
|
||||
|
||||
std::unique_ptr<Task> WonkoVersionList::remoteUpdateTask()
|
||||
{
|
||||
return std::unique_ptr<WonkoVersionListRemoteLoadTask>(new WonkoVersionListRemoteLoadTask(this, this));
|
||||
}
|
||||
std::unique_ptr<Task> WonkoVersionList::localUpdateTask()
|
||||
{
|
||||
return std::unique_ptr<WonkoVersionListLocalLoadTask>(new WonkoVersionListLocalLoadTask(this, this));
|
||||
}
|
||||
|
||||
QString WonkoVersionList::localFilename() const
|
||||
{
|
||||
return m_uid + ".json";
|
||||
}
|
||||
QJsonObject WonkoVersionList::serialized() const
|
||||
{
|
||||
return WonkoFormat::serializeVersionList(this);
|
||||
}
|
||||
|
||||
QString WonkoVersionList::humanReadable() const
|
||||
{
|
||||
return m_name.isEmpty() ? m_uid : m_name;
|
||||
}
|
||||
|
||||
bool WonkoVersionList::hasVersion(const QString &version) const
|
||||
{
|
||||
return m_lookup.contains(version);
|
||||
}
|
||||
WonkoVersionPtr WonkoVersionList::getVersion(const QString &version) const
|
||||
{
|
||||
return m_lookup.value(version);
|
||||
}
|
||||
|
||||
void WonkoVersionList::setName(const QString &name)
|
||||
{
|
||||
m_name = name;
|
||||
emit nameChanged(name);
|
||||
}
|
||||
void WonkoVersionList::setVersions(const QVector<WonkoVersionPtr> &versions)
|
||||
{
|
||||
beginResetModel();
|
||||
m_versions = versions;
|
||||
std::sort(m_versions.begin(), m_versions.end(), [](const WonkoVersionPtr &a, const WonkoVersionPtr &b)
|
||||
{
|
||||
return a->rawTime() > b->rawTime();
|
||||
});
|
||||
for (int i = 0; i < m_versions.size(); ++i)
|
||||
{
|
||||
m_lookup.insert(m_versions.at(i)->version(), m_versions.at(i));
|
||||
setupAddedVersion(i, m_versions.at(i));
|
||||
}
|
||||
|
||||
m_latest = m_versions.isEmpty() ? nullptr : m_versions.first();
|
||||
auto recommendedIt = std::find_if(m_versions.constBegin(), m_versions.constEnd(), [](const WonkoVersionPtr &ptr) { return ptr->type() == "release"; });
|
||||
m_recommended = recommendedIt == m_versions.constEnd() ? nullptr : *recommendedIt;
|
||||
endResetModel();
|
||||
}
|
||||
|
||||
void WonkoVersionList::merge(const BaseWonkoEntity::Ptr &other)
|
||||
{
|
||||
const WonkoVersionListPtr list = std::dynamic_pointer_cast<WonkoVersionList>(other);
|
||||
if (m_name != list->m_name)
|
||||
{
|
||||
setName(list->m_name);
|
||||
}
|
||||
|
||||
if (m_versions.isEmpty())
|
||||
{
|
||||
setVersions(list->m_versions);
|
||||
}
|
||||
else
|
||||
{
|
||||
for (const WonkoVersionPtr &version : list->m_versions)
|
||||
{
|
||||
if (m_lookup.contains(version->version()))
|
||||
{
|
||||
m_lookup.value(version->version())->merge(version);
|
||||
}
|
||||
else
|
||||
{
|
||||
beginInsertRows(QModelIndex(), m_versions.size(), m_versions.size());
|
||||
setupAddedVersion(m_versions.size(), version);
|
||||
m_versions.append(version);
|
||||
m_lookup.insert(version->uid(), version);
|
||||
endInsertRows();
|
||||
|
||||
if (!m_latest || version->rawTime() > m_latest->rawTime())
|
||||
{
|
||||
m_latest = version;
|
||||
emit dataChanged(index(0), index(m_versions.size() - 1), QVector<int>() << LatestRole);
|
||||
}
|
||||
if (!m_recommended || (version->type() == "release" && version->rawTime() > m_recommended->rawTime()))
|
||||
{
|
||||
m_recommended = version;
|
||||
emit dataChanged(index(0), index(m_versions.size() - 1), QVector<int>() << RecommendedRole);
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
void WonkoVersionList::setupAddedVersion(const int row, const WonkoVersionPtr &version)
|
||||
{
|
||||
connect(version.get(), &WonkoVersion::requiresChanged, this, [this, row]() { emit dataChanged(index(row), index(row), QVector<int>() << RequiresRole); });
|
||||
connect(version.get(), &WonkoVersion::timeChanged, this, [this, row]() { emit dataChanged(index(row), index(row), QVector<int>() << TimeRole << SortRole); });
|
||||
connect(version.get(), &WonkoVersion::typeChanged, this, [this, row]() { emit dataChanged(index(row), index(row), QVector<int>() << TypeRole); });
|
||||
}
|
||||
|
||||
BaseVersionPtr WonkoVersionList::getLatestStable() const
|
||||
{
|
||||
return m_latest;
|
||||
}
|
||||
BaseVersionPtr WonkoVersionList::getRecommended() const
|
||||
{
|
||||
return m_recommended;
|
||||
}
|
||||
|
||||
#include "WonkoVersionList.moc"
|
92
logic/wonko/WonkoVersionList.h
Normal file
92
logic/wonko/WonkoVersionList.h
Normal file
@ -0,0 +1,92 @@
|
||||
/* Copyright 2015 MultiMC Contributors
|
||||
*
|
||||
* Licensed under the Apache License, Version 2.0 (the "License");
|
||||
* you may not use this file except in compliance with the License.
|
||||
* You may obtain a copy of the License at
|
||||
*
|
||||
* http://www.apache.org/licenses/LICENSE-2.0
|
||||
*
|
||||
* Unless required by applicable law or agreed to in writing, software
|
||||
* distributed under the License is distributed on an "AS IS" BASIS,
|
||||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
||||
* See the License for the specific language governing permissions and
|
||||
* limitations under the License.
|
||||
*/
|
||||
|
||||
#pragma once
|
||||
|
||||
#include "BaseVersionList.h"
|
||||
#include "BaseWonkoEntity.h"
|
||||
#include <memory>
|
||||
|
||||
using WonkoVersionPtr = std::shared_ptr<class WonkoVersion>;
|
||||
using WonkoVersionListPtr = std::shared_ptr<class WonkoVersionList>;
|
||||
|
||||
class MULTIMC_LOGIC_EXPORT WonkoVersionList : public BaseVersionList, public BaseWonkoEntity
|
||||
{
|
||||
Q_OBJECT
|
||||
Q_PROPERTY(QString uid READ uid CONSTANT)
|
||||
Q_PROPERTY(QString name READ name NOTIFY nameChanged)
|
||||
public:
|
||||
explicit WonkoVersionList(const QString &uid, QObject *parent = nullptr);
|
||||
|
||||
enum Roles
|
||||
{
|
||||
UidRole = Qt::UserRole + 100,
|
||||
TimeRole,
|
||||
RequiresRole,
|
||||
WonkoVersionPtrRole
|
||||
};
|
||||
|
||||
Task *getLoadTask() override;
|
||||
bool isLoaded() override;
|
||||
const BaseVersionPtr at(int i) const override;
|
||||
int count() const override;
|
||||
void sortVersions() override;
|
||||
|
||||
BaseVersionPtr getLatestStable() const override;
|
||||
BaseVersionPtr getRecommended() const override;
|
||||
|
||||
QVariant data(const QModelIndex &index, int role) const override;
|
||||
RoleList providesRoles() const override;
|
||||
QHash<int, QByteArray> roleNames() const override;
|
||||
|
||||
std::unique_ptr<Task> remoteUpdateTask() override;
|
||||
std::unique_ptr<Task> localUpdateTask() override;
|
||||
|
||||
QString localFilename() const override;
|
||||
QJsonObject serialized() const override;
|
||||
|
||||
QString uid() const { return m_uid; }
|
||||
QString name() const { return m_name; }
|
||||
QString humanReadable() const;
|
||||
|
||||
bool hasVersion(const QString &version) const;
|
||||
WonkoVersionPtr getVersion(const QString &version) const;
|
||||
|
||||
QVector<WonkoVersionPtr> versions() const { return m_versions; }
|
||||
|
||||
public: // for usage only by parsers
|
||||
void setName(const QString &name);
|
||||
void setVersions(const QVector<WonkoVersionPtr> &versions);
|
||||
void merge(const BaseWonkoEntity::Ptr &other);
|
||||
|
||||
signals:
|
||||
void nameChanged(const QString &name);
|
||||
|
||||
protected slots:
|
||||
void updateListData(QList<BaseVersionPtr> versions) override {}
|
||||
|
||||
private:
|
||||
QVector<WonkoVersionPtr> m_versions;
|
||||
QHash<QString, WonkoVersionPtr> m_lookup;
|
||||
QString m_uid;
|
||||
QString m_name;
|
||||
|
||||
WonkoVersionPtr m_recommended;
|
||||
WonkoVersionPtr m_latest;
|
||||
|
||||
void setupAddedVersion(const int row, const WonkoVersionPtr &version);
|
||||
};
|
||||
|
||||
Q_DECLARE_METATYPE(WonkoVersionListPtr)
|
80
logic/wonko/format/WonkoFormat.cpp
Normal file
80
logic/wonko/format/WonkoFormat.cpp
Normal file
@ -0,0 +1,80 @@
|
||||
/* Copyright 2015 MultiMC Contributors
|
||||
*
|
||||
* Licensed under the Apache License, Version 2.0 (the "License");
|
||||
* you may not use this file except in compliance with the License.
|
||||
* You may obtain a copy of the License at
|
||||
*
|
||||
* http://www.apache.org/licenses/LICENSE-2.0
|
||||
*
|
||||
* Unless required by applicable law or agreed to in writing, software
|
||||
* distributed under the License is distributed on an "AS IS" BASIS,
|
||||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
||||
* See the License for the specific language governing permissions and
|
||||
* limitations under the License.
|
||||
*/
|
||||
|
||||
#include "WonkoFormat.h"
|
||||
|
||||
#include "WonkoFormatV1.h"
|
||||
|
||||
#include "wonko/WonkoIndex.h"
|
||||
#include "wonko/WonkoVersion.h"
|
||||
#include "wonko/WonkoVersionList.h"
|
||||
|
||||
static int formatVersion(const QJsonObject &obj)
|
||||
{
|
||||
if (!obj.contains("formatVersion")) {
|
||||
throw WonkoParseException(QObject::tr("Missing required field: 'formatVersion'"));
|
||||
}
|
||||
if (!obj.value("formatVersion").isDouble()) {
|
||||
throw WonkoParseException(QObject::tr("Required field has invalid type: 'formatVersion'"));
|
||||
}
|
||||
return obj.value("formatVersion").toInt();
|
||||
}
|
||||
|
||||
void WonkoFormat::parseIndex(const QJsonObject &obj, WonkoIndex *ptr)
|
||||
{
|
||||
const int version = formatVersion(obj);
|
||||
switch (version) {
|
||||
case 1:
|
||||
ptr->merge(WonkoFormatV1().parseIndexInternal(obj));
|
||||
break;
|
||||
default:
|
||||
throw WonkoParseException(QObject::tr("Unknown formatVersion: %1").arg(version));
|
||||
}
|
||||
}
|
||||
void WonkoFormat::parseVersion(const QJsonObject &obj, WonkoVersion *ptr)
|
||||
{
|
||||
const int version = formatVersion(obj);
|
||||
switch (version) {
|
||||
case 1:
|
||||
ptr->merge(WonkoFormatV1().parseVersionInternal(obj));
|
||||
break;
|
||||
default:
|
||||
throw WonkoParseException(QObject::tr("Unknown formatVersion: %1").arg(version));
|
||||
}
|
||||
}
|
||||
void WonkoFormat::parseVersionList(const QJsonObject &obj, WonkoVersionList *ptr)
|
||||
{
|
||||
const int version = formatVersion(obj);
|
||||
switch (version) {
|
||||
case 10:
|
||||
ptr->merge(WonkoFormatV1().parseVersionListInternal(obj));
|
||||
break;
|
||||
default:
|
||||
throw WonkoParseException(QObject::tr("Unknown formatVersion: %1").arg(version));
|
||||
}
|
||||
}
|
||||
|
||||
QJsonObject WonkoFormat::serializeIndex(const WonkoIndex *ptr)
|
||||
{
|
||||
return WonkoFormatV1().serializeIndexInternal(ptr);
|
||||
}
|
||||
QJsonObject WonkoFormat::serializeVersion(const WonkoVersion *ptr)
|
||||
{
|
||||
return WonkoFormatV1().serializeVersionInternal(ptr);
|
||||
}
|
||||
QJsonObject WonkoFormat::serializeVersionList(const WonkoVersionList *ptr)
|
||||
{
|
||||
return WonkoFormatV1().serializeVersionListInternal(ptr);
|
||||
}
|
54
logic/wonko/format/WonkoFormat.h
Normal file
54
logic/wonko/format/WonkoFormat.h
Normal file
@ -0,0 +1,54 @@
|
||||
/* Copyright 2015 MultiMC Contributors
|
||||
*
|
||||
* Licensed under the Apache License, Version 2.0 (the "License");
|
||||
* you may not use this file except in compliance with the License.
|
||||
* You may obtain a copy of the License at
|
||||
*
|
||||
* http://www.apache.org/licenses/LICENSE-2.0
|
||||
*
|
||||
* Unless required by applicable law or agreed to in writing, software
|
||||
* distributed under the License is distributed on an "AS IS" BASIS,
|
||||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
||||
* See the License for the specific language governing permissions and
|
||||
* limitations under the License.
|
||||
*/
|
||||
|
||||
#pragma once
|
||||
|
||||
#include <QJsonObject>
|
||||
#include <memory>
|
||||
|
||||
#include "Exception.h"
|
||||
#include "wonko/BaseWonkoEntity.h"
|
||||
|
||||
class WonkoIndex;
|
||||
class WonkoVersion;
|
||||
class WonkoVersionList;
|
||||
|
||||
class WonkoParseException : public Exception
|
||||
{
|
||||
public:
|
||||
using Exception::Exception;
|
||||
};
|
||||
|
||||
class WonkoFormat
|
||||
{
|
||||
public:
|
||||
virtual ~WonkoFormat() {}
|
||||
|
||||
static void parseIndex(const QJsonObject &obj, WonkoIndex *ptr);
|
||||
static void parseVersion(const QJsonObject &obj, WonkoVersion *ptr);
|
||||
static void parseVersionList(const QJsonObject &obj, WonkoVersionList *ptr);
|
||||
|
||||
static QJsonObject serializeIndex(const WonkoIndex *ptr);
|
||||
static QJsonObject serializeVersion(const WonkoVersion *ptr);
|
||||
static QJsonObject serializeVersionList(const WonkoVersionList *ptr);
|
||||
|
||||
protected:
|
||||
virtual BaseWonkoEntity::Ptr parseIndexInternal(const QJsonObject &obj) const = 0;
|
||||
virtual BaseWonkoEntity::Ptr parseVersionInternal(const QJsonObject &obj) const = 0;
|
||||
virtual BaseWonkoEntity::Ptr parseVersionListInternal(const QJsonObject &obj) const = 0;
|
||||
virtual QJsonObject serializeIndexInternal(const WonkoIndex *ptr) const = 0;
|
||||
virtual QJsonObject serializeVersionInternal(const WonkoVersion *ptr) const = 0;
|
||||
virtual QJsonObject serializeVersionListInternal(const WonkoVersionList *ptr) const = 0;
|
||||
};
|
156
logic/wonko/format/WonkoFormatV1.cpp
Normal file
156
logic/wonko/format/WonkoFormatV1.cpp
Normal file
@ -0,0 +1,156 @@
|
||||
/* Copyright 2015 MultiMC Contributors
|
||||
*
|
||||
* Licensed under the Apache License, Version 2.0 (the "License");
|
||||
* you may not use this file except in compliance with the License.
|
||||
* You may obtain a copy of the License at
|
||||
*
|
||||
* http://www.apache.org/licenses/LICENSE-2.0
|
||||
*
|
||||
* Unless required by applicable law or agreed to in writing, software
|
||||
* distributed under the License is distributed on an "AS IS" BASIS,
|
||||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
||||
* See the License for the specific language governing permissions and
|
||||
* limitations under the License.
|
||||
*/
|
||||
|
||||
#include "WonkoFormatV1.h"
|
||||
#include <minecraft/onesix/OneSixVersionFormat.h>
|
||||
|
||||
#include "Json.h"
|
||||
|
||||
#include "wonko/WonkoIndex.h"
|
||||
#include "wonko/WonkoVersion.h"
|
||||
#include "wonko/WonkoVersionList.h"
|
||||
#include "Env.h"
|
||||
|
||||
using namespace Json;
|
||||
|
||||
static WonkoVersionPtr parseCommonVersion(const QString &uid, const QJsonObject &obj)
|
||||
{
|
||||
const QVector<QJsonObject> requiresRaw = obj.contains("requires") ? requireIsArrayOf<QJsonObject>(obj, "requires") : QVector<QJsonObject>();
|
||||
QVector<WonkoReference> requires;
|
||||
requires.reserve(requiresRaw.size());
|
||||
std::transform(requiresRaw.begin(), requiresRaw.end(), std::back_inserter(requires), [](const QJsonObject &rObj)
|
||||
{
|
||||
WonkoReference ref(requireString(rObj, "uid"));
|
||||
ref.setVersion(ensureString(rObj, "version", QString()));
|
||||
return ref;
|
||||
});
|
||||
|
||||
WonkoVersionPtr version = std::make_shared<WonkoVersion>(uid, requireString(obj, "version"));
|
||||
if (obj.value("time").isString())
|
||||
{
|
||||
version->setTime(QDateTime::fromString(requireString(obj, "time"), Qt::ISODate).toMSecsSinceEpoch() / 1000);
|
||||
}
|
||||
else
|
||||
{
|
||||
version->setTime(requireInteger(obj, "time"));
|
||||
}
|
||||
version->setType(ensureString(obj, "type", QString()));
|
||||
version->setRequires(requires);
|
||||
return version;
|
||||
}
|
||||
static void serializeCommonVersion(const WonkoVersion *version, QJsonObject &obj)
|
||||
{
|
||||
QJsonArray requires;
|
||||
for (const WonkoReference &ref : version->requires())
|
||||
{
|
||||
if (ref.version().isEmpty())
|
||||
{
|
||||
requires.append(QJsonObject({{"uid", ref.uid()}}));
|
||||
}
|
||||
else
|
||||
{
|
||||
requires.append(QJsonObject({
|
||||
{"uid", ref.uid()},
|
||||
{"version", ref.version()}
|
||||
}));
|
||||
}
|
||||
}
|
||||
|
||||
obj.insert("version", version->version());
|
||||
obj.insert("type", version->type());
|
||||
obj.insert("time", version->time().toString(Qt::ISODate));
|
||||
obj.insert("requires", requires);
|
||||
}
|
||||
|
||||
BaseWonkoEntity::Ptr WonkoFormatV1::parseIndexInternal(const QJsonObject &obj) const
|
||||
{
|
||||
const QVector<QJsonObject> objects = requireIsArrayOf<QJsonObject>(obj, "index");
|
||||
QVector<WonkoVersionListPtr> lists;
|
||||
lists.reserve(objects.size());
|
||||
std::transform(objects.begin(), objects.end(), std::back_inserter(lists), [](const QJsonObject &obj)
|
||||
{
|
||||
WonkoVersionListPtr list = std::make_shared<WonkoVersionList>(requireString(obj, "uid"));
|
||||
list->setName(ensureString(obj, "name", QString()));
|
||||
return list;
|
||||
});
|
||||
return std::make_shared<WonkoIndex>(lists);
|
||||
}
|
||||
BaseWonkoEntity::Ptr WonkoFormatV1::parseVersionInternal(const QJsonObject &obj) const
|
||||
{
|
||||
WonkoVersionPtr version = parseCommonVersion(requireString(obj, "uid"), obj);
|
||||
|
||||
version->setData(OneSixVersionFormat::versionFileFromJson(QJsonDocument(obj),
|
||||
QString("%1/%2.json").arg(version->uid(), version->version()),
|
||||
obj.contains("order")));
|
||||
return version;
|
||||
}
|
||||
BaseWonkoEntity::Ptr WonkoFormatV1::parseVersionListInternal(const QJsonObject &obj) const
|
||||
{
|
||||
const QString uid = requireString(obj, "uid");
|
||||
|
||||
const QVector<QJsonObject> versionsRaw = requireIsArrayOf<QJsonObject>(obj, "versions");
|
||||
QVector<WonkoVersionPtr> versions;
|
||||
versions.reserve(versionsRaw.size());
|
||||
std::transform(versionsRaw.begin(), versionsRaw.end(), std::back_inserter(versions), [this, uid](const QJsonObject &vObj)
|
||||
{ return parseCommonVersion(uid, vObj); });
|
||||
|
||||
WonkoVersionListPtr list = std::make_shared<WonkoVersionList>(uid);
|
||||
list->setName(ensureString(obj, "name", QString()));
|
||||
list->setVersions(versions);
|
||||
return list;
|
||||
}
|
||||
|
||||
QJsonObject WonkoFormatV1::serializeIndexInternal(const WonkoIndex *ptr) const
|
||||
{
|
||||
QJsonArray index;
|
||||
for (const WonkoVersionListPtr &list : ptr->lists())
|
||||
{
|
||||
index.append(QJsonObject({
|
||||
{"uid", list->uid()},
|
||||
{"name", list->name()}
|
||||
}));
|
||||
}
|
||||
return QJsonObject({
|
||||
{"formatVersion", 1},
|
||||
{"index", index}
|
||||
});
|
||||
}
|
||||
QJsonObject WonkoFormatV1::serializeVersionInternal(const WonkoVersion *ptr) const
|
||||
{
|
||||
QJsonObject obj = OneSixVersionFormat::versionFileToJson(ptr->data(), true).object();
|
||||
serializeCommonVersion(ptr, obj);
|
||||
obj.insert("formatVersion", 1);
|
||||
obj.insert("uid", ptr->uid());
|
||||
// TODO: the name should be looked up in the UI based on the uid
|
||||
obj.insert("name", ENV.wonkoIndex()->getListGuaranteed(ptr->uid())->name());
|
||||
|
||||
return obj;
|
||||
}
|
||||
QJsonObject WonkoFormatV1::serializeVersionListInternal(const WonkoVersionList *ptr) const
|
||||
{
|
||||
QJsonArray versions;
|
||||
for (const WonkoVersionPtr &version : ptr->versions())
|
||||
{
|
||||
QJsonObject obj;
|
||||
serializeCommonVersion(version.get(), obj);
|
||||
versions.append(obj);
|
||||
}
|
||||
return QJsonObject({
|
||||
{"formatVersion", 10},
|
||||
{"uid", ptr->uid()},
|
||||
{"name", ptr->name().isNull() ? QJsonValue() : ptr->name()},
|
||||
{"versions", versions}
|
||||
});
|
||||
}
|
30
logic/wonko/format/WonkoFormatV1.h
Normal file
30
logic/wonko/format/WonkoFormatV1.h
Normal file
@ -0,0 +1,30 @@
|
||||
/* Copyright 2015 MultiMC Contributors
|
||||
*
|
||||
* Licensed under the Apache License, Version 2.0 (the "License");
|
||||
* you may not use this file except in compliance with the License.
|
||||
* You may obtain a copy of the License at
|
||||
*
|
||||
* http://www.apache.org/licenses/LICENSE-2.0
|
||||
*
|
||||
* Unless required by applicable law or agreed to in writing, software
|
||||
* distributed under the License is distributed on an "AS IS" BASIS,
|
||||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
||||
* See the License for the specific language governing permissions and
|
||||
* limitations under the License.
|
||||
*/
|
||||
|
||||
#pragma once
|
||||
|
||||
#include "WonkoFormat.h"
|
||||
|
||||
class WonkoFormatV1 : public WonkoFormat
|
||||
{
|
||||
public:
|
||||
BaseWonkoEntity::Ptr parseIndexInternal(const QJsonObject &obj) const override;
|
||||
BaseWonkoEntity::Ptr parseVersionInternal(const QJsonObject &obj) const override;
|
||||
BaseWonkoEntity::Ptr parseVersionListInternal(const QJsonObject &obj) const override;
|
||||
|
||||
QJsonObject serializeIndexInternal(const WonkoIndex *ptr) const override;
|
||||
QJsonObject serializeVersionInternal(const WonkoVersion *ptr) const override;
|
||||
QJsonObject serializeVersionListInternal(const WonkoVersionList *ptr) const override;
|
||||
};
|
117
logic/wonko/tasks/BaseWonkoEntityLocalLoadTask.cpp
Normal file
117
logic/wonko/tasks/BaseWonkoEntityLocalLoadTask.cpp
Normal file
@ -0,0 +1,117 @@
|
||||
/* Copyright 2015 MultiMC Contributors
|
||||
*
|
||||
* Licensed under the Apache License, Version 2.0 (the "License");
|
||||
* you may not use this file except in compliance with the License.
|
||||
* You may obtain a copy of the License at
|
||||
*
|
||||
* http://www.apache.org/licenses/LICENSE-2.0
|
||||
*
|
||||
* Unless required by applicable law or agreed to in writing, software
|
||||
* distributed under the License is distributed on an "AS IS" BASIS,
|
||||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
||||
* See the License for the specific language governing permissions and
|
||||
* limitations under the License.
|
||||
*/
|
||||
|
||||
#include "BaseWonkoEntityLocalLoadTask.h"
|
||||
|
||||
#include <QFile>
|
||||
|
||||
#include "wonko/format/WonkoFormat.h"
|
||||
#include "wonko/WonkoUtil.h"
|
||||
#include "wonko/WonkoIndex.h"
|
||||
#include "wonko/WonkoVersion.h"
|
||||
#include "wonko/WonkoVersionList.h"
|
||||
#include "Env.h"
|
||||
#include "Json.h"
|
||||
|
||||
BaseWonkoEntityLocalLoadTask::BaseWonkoEntityLocalLoadTask(BaseWonkoEntity *entity, QObject *parent)
|
||||
: Task(parent), m_entity(entity)
|
||||
{
|
||||
}
|
||||
|
||||
void BaseWonkoEntityLocalLoadTask::executeTask()
|
||||
{
|
||||
const QString fname = Wonko::localWonkoDir().absoluteFilePath(filename());
|
||||
if (!QFile::exists(fname))
|
||||
{
|
||||
emitFailed(tr("File doesn't exist"));
|
||||
return;
|
||||
}
|
||||
|
||||
setStatus(tr("Reading %1...").arg(name()));
|
||||
setProgress(0, 0);
|
||||
|
||||
try
|
||||
{
|
||||
parse(Json::requireObject(Json::requireDocument(fname, name()), name()));
|
||||
m_entity->notifyLocalLoadComplete();
|
||||
emitSucceeded();
|
||||
}
|
||||
catch (Exception &e)
|
||||
{
|
||||
emitFailed(tr("Unable to parse file %1: %2").arg(fname, e.cause()));
|
||||
}
|
||||
}
|
||||
|
||||
// WONKO INDEX //
|
||||
WonkoIndexLocalLoadTask::WonkoIndexLocalLoadTask(WonkoIndex *index, QObject *parent)
|
||||
: BaseWonkoEntityLocalLoadTask(index, parent)
|
||||
{
|
||||
}
|
||||
QString WonkoIndexLocalLoadTask::filename() const
|
||||
{
|
||||
return "index.json";
|
||||
}
|
||||
QString WonkoIndexLocalLoadTask::name() const
|
||||
{
|
||||
return tr("Wonko Index");
|
||||
}
|
||||
void WonkoIndexLocalLoadTask::parse(const QJsonObject &obj) const
|
||||
{
|
||||
WonkoFormat::parseIndex(obj, dynamic_cast<WonkoIndex *>(entity()));
|
||||
}
|
||||
|
||||
// WONKO VERSION LIST //
|
||||
WonkoVersionListLocalLoadTask::WonkoVersionListLocalLoadTask(WonkoVersionList *list, QObject *parent)
|
||||
: BaseWonkoEntityLocalLoadTask(list, parent)
|
||||
{
|
||||
}
|
||||
QString WonkoVersionListLocalLoadTask::filename() const
|
||||
{
|
||||
return list()->uid() + ".json";
|
||||
}
|
||||
QString WonkoVersionListLocalLoadTask::name() const
|
||||
{
|
||||
return tr("Wonko Version List for %1").arg(list()->humanReadable());
|
||||
}
|
||||
void WonkoVersionListLocalLoadTask::parse(const QJsonObject &obj) const
|
||||
{
|
||||
WonkoFormat::parseVersionList(obj, list());
|
||||
}
|
||||
WonkoVersionList *WonkoVersionListLocalLoadTask::list() const
|
||||
{
|
||||
return dynamic_cast<WonkoVersionList *>(entity());
|
||||
}
|
||||
|
||||
// WONKO VERSION //
|
||||
WonkoVersionLocalLoadTask::WonkoVersionLocalLoadTask(WonkoVersion *version, QObject *parent)
|
||||
: BaseWonkoEntityLocalLoadTask(version, parent)
|
||||
{
|
||||
}
|
||||
QString WonkoVersionLocalLoadTask::filename() const
|
||||
{
|
||||
return version()->uid() + "/" + version()->version() + ".json";
|
||||
}
|
||||
QString WonkoVersionLocalLoadTask::name() const
|
||||
{
|
||||
return tr("Wonko Version for %1").arg(version()->name());
|
||||
}
|
||||
void WonkoVersionLocalLoadTask::parse(const QJsonObject &obj) const
|
||||
{
|
||||
WonkoFormat::parseVersion(obj, version());
|
||||
}
|
||||
WonkoVersion *WonkoVersionLocalLoadTask::version() const
|
||||
{
|
||||
return dynamic_cast<WonkoVersion *>(entity());
|
||||
}
|
81
logic/wonko/tasks/BaseWonkoEntityLocalLoadTask.h
Normal file
81
logic/wonko/tasks/BaseWonkoEntityLocalLoadTask.h
Normal file
@ -0,0 +1,81 @@
|
||||
/* Copyright 2015 MultiMC Contributors
|
||||
*
|
||||
* Licensed under the Apache License, Version 2.0 (the "License");
|
||||
* you may not use this file except in compliance with the License.
|
||||
* You may obtain a copy of the License at
|
||||
*
|
||||
* http://www.apache.org/licenses/LICENSE-2.0
|
||||
*
|
||||
* Unless required by applicable law or agreed to in writing, software
|
||||
* distributed under the License is distributed on an "AS IS" BASIS,
|
||||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
||||
* See the License for the specific language governing permissions and
|
||||
* limitations under the License.
|
||||
*/
|
||||
|
||||
#pragma once
|
||||
|
||||
#include "tasks/Task.h"
|
||||
#include <memory>
|
||||
|
||||
class BaseWonkoEntity;
|
||||
class WonkoIndex;
|
||||
class WonkoVersionList;
|
||||
class WonkoVersion;
|
||||
|
||||
class BaseWonkoEntityLocalLoadTask : public Task
|
||||
{
|
||||
Q_OBJECT
|
||||
public:
|
||||
explicit BaseWonkoEntityLocalLoadTask(BaseWonkoEntity *entity, QObject *parent = nullptr);
|
||||
|
||||
protected:
|
||||
virtual QString filename() const = 0;
|
||||
virtual QString name() const = 0;
|
||||
virtual void parse(const QJsonObject &obj) const = 0;
|
||||
|
||||
BaseWonkoEntity *entity() const { return m_entity; }
|
||||
|
||||
private:
|
||||
void executeTask() override;
|
||||
|
||||
BaseWonkoEntity *m_entity;
|
||||
};
|
||||
|
||||
class WonkoIndexLocalLoadTask : public BaseWonkoEntityLocalLoadTask
|
||||
{
|
||||
Q_OBJECT
|
||||
public:
|
||||
explicit WonkoIndexLocalLoadTask(WonkoIndex *index, QObject *parent = nullptr);
|
||||
|
||||
private:
|
||||
QString filename() const override;
|
||||
QString name() const override;
|
||||
void parse(const QJsonObject &obj) const override;
|
||||
};
|
||||
class WonkoVersionListLocalLoadTask : public BaseWonkoEntityLocalLoadTask
|
||||
{
|
||||
Q_OBJECT
|
||||
public:
|
||||
explicit WonkoVersionListLocalLoadTask(WonkoVersionList *list, QObject *parent = nullptr);
|
||||
|
||||
private:
|
||||
QString filename() const override;
|
||||
QString name() const override;
|
||||
void parse(const QJsonObject &obj) const override;
|
||||
|
||||
WonkoVersionList *list() const;
|
||||
};
|
||||
class WonkoVersionLocalLoadTask : public BaseWonkoEntityLocalLoadTask
|
||||
{
|
||||
Q_OBJECT
|
||||
public:
|
||||
explicit WonkoVersionLocalLoadTask(WonkoVersion *version, QObject *parent = nullptr);
|
||||
|
||||
private:
|
||||
QString filename() const override;
|
||||
QString name() const override;
|
||||
void parse(const QJsonObject &obj) const override;
|
||||
|
||||
WonkoVersion *version() const;
|
||||
};
|
126
logic/wonko/tasks/BaseWonkoEntityRemoteLoadTask.cpp
Normal file
126
logic/wonko/tasks/BaseWonkoEntityRemoteLoadTask.cpp
Normal file
@ -0,0 +1,126 @@
|
||||
/* Copyright 2015 MultiMC Contributors
|
||||
*
|
||||
* Licensed under the Apache License, Version 2.0 (the "License");
|
||||
* you may not use this file except in compliance with the License.
|
||||
* You may obtain a copy of the License at
|
||||
*
|
||||
* http://www.apache.org/licenses/LICENSE-2.0
|
||||
*
|
||||
* Unless required by applicable law or agreed to in writing, software
|
||||
* distributed under the License is distributed on an "AS IS" BASIS,
|
||||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
||||
* See the License for the specific language governing permissions and
|
||||
* limitations under the License.
|
||||
*/
|
||||
|
||||
#include "BaseWonkoEntityRemoteLoadTask.h"
|
||||
|
||||
#include "net/CacheDownload.h"
|
||||
#include "net/HttpMetaCache.h"
|
||||
#include "net/NetJob.h"
|
||||
#include "wonko/format/WonkoFormat.h"
|
||||
#include "wonko/WonkoUtil.h"
|
||||
#include "wonko/WonkoIndex.h"
|
||||
#include "wonko/WonkoVersion.h"
|
||||
#include "wonko/WonkoVersionList.h"
|
||||
#include "Env.h"
|
||||
#include "Json.h"
|
||||
|
||||
BaseWonkoEntityRemoteLoadTask::BaseWonkoEntityRemoteLoadTask(BaseWonkoEntity *entity, QObject *parent)
|
||||
: Task(parent), m_entity(entity)
|
||||
{
|
||||
}
|
||||
|
||||
void BaseWonkoEntityRemoteLoadTask::executeTask()
|
||||
{
|
||||
NetJob *job = new NetJob(name());
|
||||
|
||||
auto entry = ENV.metacache()->resolveEntry("wonko", url().toString());
|
||||
entry->setStale(true);
|
||||
m_dl = CacheDownload::make(url(), entry);
|
||||
job->addNetAction(m_dl);
|
||||
connect(job, &NetJob::failed, this, &BaseWonkoEntityRemoteLoadTask::emitFailed);
|
||||
connect(job, &NetJob::succeeded, this, &BaseWonkoEntityRemoteLoadTask::networkFinished);
|
||||
connect(job, &NetJob::status, this, &BaseWonkoEntityRemoteLoadTask::setStatus);
|
||||
connect(job, &NetJob::progress, this, &BaseWonkoEntityRemoteLoadTask::setProgress);
|
||||
job->start();
|
||||
}
|
||||
|
||||
void BaseWonkoEntityRemoteLoadTask::networkFinished()
|
||||
{
|
||||
setStatus(tr("Parsing..."));
|
||||
setProgress(0, 0);
|
||||
|
||||
try
|
||||
{
|
||||
parse(Json::requireObject(Json::requireDocument(m_dl->getTargetFilepath(), name()), name()));
|
||||
m_entity->notifyRemoteLoadComplete();
|
||||
emitSucceeded();
|
||||
}
|
||||
catch (Exception &e)
|
||||
{
|
||||
emitFailed(tr("Unable to parse response: %1").arg(e.cause()));
|
||||
}
|
||||
}
|
||||
|
||||
// WONKO INDEX //
|
||||
WonkoIndexRemoteLoadTask::WonkoIndexRemoteLoadTask(WonkoIndex *index, QObject *parent)
|
||||
: BaseWonkoEntityRemoteLoadTask(index, parent)
|
||||
{
|
||||
}
|
||||
QUrl WonkoIndexRemoteLoadTask::url() const
|
||||
{
|
||||
return Wonko::indexUrl();
|
||||
}
|
||||
QString WonkoIndexRemoteLoadTask::name() const
|
||||
{
|
||||
return tr("Wonko Index");
|
||||
}
|
||||
void WonkoIndexRemoteLoadTask::parse(const QJsonObject &obj) const
|
||||
{
|
||||
WonkoFormat::parseIndex(obj, dynamic_cast<WonkoIndex *>(entity()));
|
||||
}
|
||||
|
||||
// WONKO VERSION LIST //
|
||||
WonkoVersionListRemoteLoadTask::WonkoVersionListRemoteLoadTask(WonkoVersionList *list, QObject *parent)
|
||||
: BaseWonkoEntityRemoteLoadTask(list, parent)
|
||||
{
|
||||
}
|
||||
QUrl WonkoVersionListRemoteLoadTask::url() const
|
||||
{
|
||||
return Wonko::versionListUrl(list()->uid());
|
||||
}
|
||||
QString WonkoVersionListRemoteLoadTask::name() const
|
||||
{
|
||||
return tr("Wonko Version List for %1").arg(list()->humanReadable());
|
||||
}
|
||||
void WonkoVersionListRemoteLoadTask::parse(const QJsonObject &obj) const
|
||||
{
|
||||
WonkoFormat::parseVersionList(obj, list());
|
||||
}
|
||||
WonkoVersionList *WonkoVersionListRemoteLoadTask::list() const
|
||||
{
|
||||
return dynamic_cast<WonkoVersionList *>(entity());
|
||||
}
|
||||
|
||||
// WONKO VERSION //
|
||||
WonkoVersionRemoteLoadTask::WonkoVersionRemoteLoadTask(WonkoVersion *version, QObject *parent)
|
||||
: BaseWonkoEntityRemoteLoadTask(version, parent)
|
||||
{
|
||||
}
|
||||
QUrl WonkoVersionRemoteLoadTask::url() const
|
||||
{
|
||||
return Wonko::versionUrl(version()->uid(), version()->version());
|
||||
}
|
||||
QString WonkoVersionRemoteLoadTask::name() const
|
||||
{
|
||||
return tr("Wonko Version for %1").arg(version()->name());
|
||||
}
|
||||
void WonkoVersionRemoteLoadTask::parse(const QJsonObject &obj) const
|
||||
{
|
||||
WonkoFormat::parseVersion(obj, version());
|
||||
}
|
||||
WonkoVersion *WonkoVersionRemoteLoadTask::version() const
|
||||
{
|
||||
return dynamic_cast<WonkoVersion *>(entity());
|
||||
}
|
85
logic/wonko/tasks/BaseWonkoEntityRemoteLoadTask.h
Normal file
85
logic/wonko/tasks/BaseWonkoEntityRemoteLoadTask.h
Normal file
@ -0,0 +1,85 @@
|
||||
/* Copyright 2015 MultiMC Contributors
|
||||
*
|
||||
* Licensed under the Apache License, Version 2.0 (the "License");
|
||||
* you may not use this file except in compliance with the License.
|
||||
* You may obtain a copy of the License at
|
||||
*
|
||||
* http://www.apache.org/licenses/LICENSE-2.0
|
||||
*
|
||||
* Unless required by applicable law or agreed to in writing, software
|
||||
* distributed under the License is distributed on an "AS IS" BASIS,
|
||||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
||||
* See the License for the specific language governing permissions and
|
||||
* limitations under the License.
|
||||
*/
|
||||
|
||||
#pragma once
|
||||
|
||||
#include "tasks/Task.h"
|
||||
#include <memory>
|
||||
|
||||
class BaseWonkoEntity;
|
||||
class WonkoIndex;
|
||||
class WonkoVersionList;
|
||||
class WonkoVersion;
|
||||
|
||||
class BaseWonkoEntityRemoteLoadTask : public Task
|
||||
{
|
||||
Q_OBJECT
|
||||
public:
|
||||
explicit BaseWonkoEntityRemoteLoadTask(BaseWonkoEntity *entity, QObject *parent = nullptr);
|
||||
|
||||
protected:
|
||||
virtual QUrl url() const = 0;
|
||||
virtual QString name() const = 0;
|
||||
virtual void parse(const QJsonObject &obj) const = 0;
|
||||
|
||||
BaseWonkoEntity *entity() const { return m_entity; }
|
||||
|
||||
private slots:
|
||||
void networkFinished();
|
||||
|
||||
private:
|
||||
void executeTask() override;
|
||||
|
||||
BaseWonkoEntity *m_entity;
|
||||
std::shared_ptr<class CacheDownload> m_dl;
|
||||
};
|
||||
|
||||
class WonkoIndexRemoteLoadTask : public BaseWonkoEntityRemoteLoadTask
|
||||
{
|
||||
Q_OBJECT
|
||||
public:
|
||||
explicit WonkoIndexRemoteLoadTask(WonkoIndex *index, QObject *parent = nullptr);
|
||||
|
||||
private:
|
||||
QUrl url() const override;
|
||||
QString name() const override;
|
||||
void parse(const QJsonObject &obj) const override;
|
||||
};
|
||||
class WonkoVersionListRemoteLoadTask : public BaseWonkoEntityRemoteLoadTask
|
||||
{
|
||||
Q_OBJECT
|
||||
public:
|
||||
explicit WonkoVersionListRemoteLoadTask(WonkoVersionList *list, QObject *parent = nullptr);
|
||||
|
||||
private:
|
||||
QUrl url() const override;
|
||||
QString name() const override;
|
||||
void parse(const QJsonObject &obj) const override;
|
||||
|
||||
WonkoVersionList *list() const;
|
||||
};
|
||||
class WonkoVersionRemoteLoadTask : public BaseWonkoEntityRemoteLoadTask
|
||||
{
|
||||
Q_OBJECT
|
||||
public:
|
||||
explicit WonkoVersionRemoteLoadTask(WonkoVersion *version, QObject *parent = nullptr);
|
||||
|
||||
private:
|
||||
QUrl url() const override;
|
||||
QString name() const override;
|
||||
void parse(const QJsonObject &obj) const override;
|
||||
|
||||
WonkoVersion *version() const;
|
||||
};
|
@ -37,6 +37,10 @@ add_unit_test(GZip tst_GZip.cpp)
|
||||
add_unit_test(JavaVersion tst_JavaVersion.cpp)
|
||||
add_unit_test(ParseUtils tst_ParseUtils.cpp)
|
||||
add_unit_test(MojangVersionFormat tst_MojangVersionFormat.cpp)
|
||||
add_unit_test(BaseWonkoEntityLocalLoadTask tst_BaseWonkoEntityLocalLoadTask.cpp)
|
||||
add_unit_test(BaseWonkoEntityRemoteLoadTask tst_BaseWonkoEntityRemoteLoadTask.cpp)
|
||||
add_unit_test(WonkoVersionList tst_WonkoVersionList.cpp)
|
||||
add_unit_test(WonkoIndex tst_WonkoIndex.cpp)
|
||||
|
||||
# Tests END #
|
||||
|
||||
|
15
tests/tst_BaseWonkoEntityLocalLoadTask.cpp
Normal file
15
tests/tst_BaseWonkoEntityLocalLoadTask.cpp
Normal file
@ -0,0 +1,15 @@
|
||||
#include <QTest>
|
||||
#include "TestUtil.h"
|
||||
|
||||
#include "wonko/tasks/BaseWonkoEntityLocalLoadTask.h"
|
||||
|
||||
class BaseWonkoEntityLocalLoadTaskTest : public QObject
|
||||
{
|
||||
Q_OBJECT
|
||||
private
|
||||
slots:
|
||||
};
|
||||
|
||||
QTEST_GUILESS_MAIN(BaseWonkoEntityLocalLoadTaskTest)
|
||||
|
||||
#include "tst_BaseWonkoEntityLocalLoadTask.moc"
|
15
tests/tst_BaseWonkoEntityRemoteLoadTask.cpp
Normal file
15
tests/tst_BaseWonkoEntityRemoteLoadTask.cpp
Normal file
@ -0,0 +1,15 @@
|
||||
#include <QTest>
|
||||
#include "TestUtil.h"
|
||||
|
||||
#include "wonko/tasks/BaseWonkoEntityRemoteLoadTask.h"
|
||||
|
||||
class BaseWonkoEntityRemoteLoadTaskTest : public QObject
|
||||
{
|
||||
Q_OBJECT
|
||||
private
|
||||
slots:
|
||||
};
|
||||
|
||||
QTEST_GUILESS_MAIN(BaseWonkoEntityRemoteLoadTaskTest)
|
||||
|
||||
#include "tst_BaseWonkoEntityRemoteLoadTask.moc"
|
50
tests/tst_WonkoIndex.cpp
Normal file
50
tests/tst_WonkoIndex.cpp
Normal file
@ -0,0 +1,50 @@
|
||||
#include <QTest>
|
||||
#include "TestUtil.h"
|
||||
|
||||
#include "wonko/WonkoIndex.h"
|
||||
#include "wonko/WonkoVersionList.h"
|
||||
#include "Env.h"
|
||||
|
||||
class WonkoIndexTest : public QObject
|
||||
{
|
||||
Q_OBJECT
|
||||
private
|
||||
slots:
|
||||
void test_isProvidedByEnv()
|
||||
{
|
||||
QVERIFY(ENV.wonkoIndex() != nullptr);
|
||||
QCOMPARE(ENV.wonkoIndex(), ENV.wonkoIndex());
|
||||
}
|
||||
|
||||
void test_providesTasks()
|
||||
{
|
||||
QVERIFY(ENV.wonkoIndex()->localUpdateTask() != nullptr);
|
||||
QVERIFY(ENV.wonkoIndex()->remoteUpdateTask() != nullptr);
|
||||
}
|
||||
|
||||
void test_hasUid_and_getList()
|
||||
{
|
||||
WonkoIndex windex({std::make_shared<WonkoVersionList>("list1"), std::make_shared<WonkoVersionList>("list2"), std::make_shared<WonkoVersionList>("list3")});
|
||||
QVERIFY(windex.hasUid("list1"));
|
||||
QVERIFY(!windex.hasUid("asdf"));
|
||||
QVERIFY(windex.getList("list2") != nullptr);
|
||||
QCOMPARE(windex.getList("list2")->uid(), QString("list2"));
|
||||
QVERIFY(windex.getList("adsf") == nullptr);
|
||||
}
|
||||
|
||||
void test_merge()
|
||||
{
|
||||
WonkoIndex windex({std::make_shared<WonkoVersionList>("list1"), std::make_shared<WonkoVersionList>("list2"), std::make_shared<WonkoVersionList>("list3")});
|
||||
QCOMPARE(windex.lists().size(), 3);
|
||||
windex.merge(std::shared_ptr<WonkoIndex>(new WonkoIndex({std::make_shared<WonkoVersionList>("list1"), std::make_shared<WonkoVersionList>("list2"), std::make_shared<WonkoVersionList>("list3")})));
|
||||
QCOMPARE(windex.lists().size(), 3);
|
||||
windex.merge(std::shared_ptr<WonkoIndex>(new WonkoIndex({std::make_shared<WonkoVersionList>("list4"), std::make_shared<WonkoVersionList>("list2"), std::make_shared<WonkoVersionList>("list5")})));
|
||||
QCOMPARE(windex.lists().size(), 5);
|
||||
windex.merge(std::shared_ptr<WonkoIndex>(new WonkoIndex({std::make_shared<WonkoVersionList>("list6")})));
|
||||
QCOMPARE(windex.lists().size(), 6);
|
||||
}
|
||||
};
|
||||
|
||||
QTEST_GUILESS_MAIN(WonkoIndexTest)
|
||||
|
||||
#include "tst_WonkoIndex.moc"
|
15
tests/tst_WonkoVersionList.cpp
Normal file
15
tests/tst_WonkoVersionList.cpp
Normal file
@ -0,0 +1,15 @@
|
||||
#include <QTest>
|
||||
#include "TestUtil.h"
|
||||
|
||||
#include "wonko/WonkoVersionList.h"
|
||||
|
||||
class WonkoVersionListTest : public QObject
|
||||
{
|
||||
Q_OBJECT
|
||||
private
|
||||
slots:
|
||||
};
|
||||
|
||||
QTEST_GUILESS_MAIN(WonkoVersionListTest)
|
||||
|
||||
#include "tst_WonkoVersionList.moc"
|
Reference in New Issue
Block a user