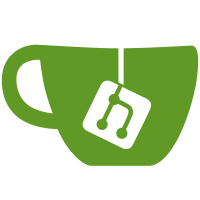
This aims to continue decoupling other types of resources (e.g. resource packs, shader packs, etc) from mods, so that we don't have to continuously watch our backs for changes to one of them affecting the others. To do so, this creates a more general list model for resources, based on the mods one, that allows you to extend it with functionality for other resources. I had to do some template and preprocessor stuff to get around the QObject limitation of not allowing templated classes, so that's sadge :c On the other hand, I tried cleaning up most general-purpose code in the mod model, and added some documentation, because it looks nice :D Signed-off-by: flow <flowlnlnln@gmail.com>
39 lines
756 B
C++
39 lines
756 B
C++
#pragma once
|
|
|
|
#include <QDebug>
|
|
#include <QObject>
|
|
|
|
#include "minecraft/mod/Mod.h"
|
|
#include "minecraft/mod/ModDetails.h"
|
|
|
|
#include "tasks/Task.h"
|
|
|
|
class LocalModParseTask : public Task
|
|
{
|
|
Q_OBJECT
|
|
public:
|
|
struct Result {
|
|
std::shared_ptr<ModDetails> details;
|
|
};
|
|
using ResultPtr = std::shared_ptr<Result>;
|
|
ResultPtr result() const {
|
|
return m_result;
|
|
}
|
|
|
|
LocalModParseTask(int token, ResourceType type, const QFileInfo & modFile);
|
|
void executeTask() override;
|
|
|
|
[[nodiscard]] int token() const { return m_token; }
|
|
|
|
private:
|
|
void processAsZip();
|
|
void processAsFolder();
|
|
void processAsLitemod();
|
|
|
|
private:
|
|
int m_token;
|
|
ResourceType m_type;
|
|
QFileInfo m_modFile;
|
|
ResultPtr m_result;
|
|
};
|