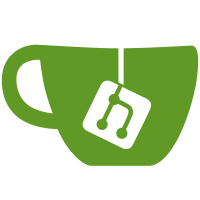
While implementing the resource pack downloader in another branch, I noticed that most of the code in the success callback was identical in both cases, safe for a few minute differences in strings. So, this tries to make it easier to share this piece of code. However, it still leaves the possibility of extending the methods in ResourceModel to accomodate for cases where this similarity may not hold. Signed-off-by: flow <flowlnlnln@gmail.com>
67 lines
1.8 KiB
C++
67 lines
1.8 KiB
C++
#include "ModModel.h"
|
|
|
|
#include "minecraft/MinecraftInstance.h"
|
|
#include "minecraft/PackProfile.h"
|
|
|
|
#include <QMessageBox>
|
|
|
|
namespace ResourceDownload {
|
|
|
|
ModModel::ModModel(BaseInstance const& base_inst, ResourceAPI* api) : ResourceModel(base_inst, api) {}
|
|
|
|
/******** Make data requests ********/
|
|
|
|
ResourceAPI::SearchArgs ModModel::createSearchArguments()
|
|
{
|
|
auto profile = static_cast<MinecraftInstance const&>(m_base_instance).getPackProfile();
|
|
|
|
Q_ASSERT(profile);
|
|
Q_ASSERT(m_filter);
|
|
|
|
std::optional<std::list<Version>> versions{};
|
|
|
|
{ // Version filter
|
|
if (!m_filter->versions.empty())
|
|
versions = m_filter->versions;
|
|
}
|
|
|
|
auto sort = getCurrentSortingMethodByIndex();
|
|
|
|
return { ModPlatform::ResourceType::MOD, m_next_search_offset, m_search_term, sort, profile->getModLoaders(), versions };
|
|
}
|
|
|
|
ResourceAPI::VersionSearchArgs ModModel::createVersionsArguments(QModelIndex& entry)
|
|
{
|
|
auto& pack = m_packs[entry.row()];
|
|
auto profile = static_cast<MinecraftInstance const&>(m_base_instance).getPackProfile();
|
|
|
|
Q_ASSERT(profile);
|
|
Q_ASSERT(m_filter);
|
|
|
|
std::optional<std::list<Version>> versions{};
|
|
if (!m_filter->versions.empty())
|
|
versions = m_filter->versions;
|
|
|
|
return { pack, versions, profile->getModLoaders() };
|
|
}
|
|
|
|
ResourceAPI::ProjectInfoArgs ModModel::createInfoArguments(QModelIndex& entry)
|
|
{
|
|
auto& pack = m_packs[entry.row()];
|
|
return { pack };
|
|
}
|
|
|
|
void ModModel::searchWithTerm(const QString& term, unsigned int sort, bool filter_changed)
|
|
{
|
|
if (m_search_term == term && m_search_term.isNull() == term.isNull() && m_current_sort_index == sort && !filter_changed) {
|
|
return;
|
|
}
|
|
|
|
setSearchTerm(term);
|
|
m_current_sort_index = sort;
|
|
|
|
refresh();
|
|
}
|
|
|
|
} // namespace ResourceDownload
|