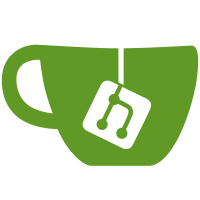
This task is responsible for checking if the mod has metadata for a specific provider, and create it if it doesn't. In the context of the mod updater, this is not the best architecture, since we do a single task for each mod. However, this way of structuring it allows us to use it later on in more diverse scenarios. This way we decouple this task from the mod updater, trading off some performance (though that will be mitigated when we have a way of running arbitrary tasks concurrently). Signed-off-by: flow <flowlnlnln@gmail.com>
42 lines
862 B
C++
42 lines
862 B
C++
#pragma once
|
|
|
|
#include "ModIndex.h"
|
|
#include "tasks/SequentialTask.h"
|
|
|
|
class Mod;
|
|
class QDir;
|
|
class MultipleOptionsTask;
|
|
|
|
class EnsureMetadataTask : public Task {
|
|
Q_OBJECT
|
|
|
|
public:
|
|
EnsureMetadataTask(Mod&, QDir&, bool try_all, ModPlatform::Provider = ModPlatform::Provider::MODRINTH);
|
|
|
|
public slots:
|
|
bool abort() override;
|
|
protected slots:
|
|
void executeTask() override;
|
|
|
|
private:
|
|
// FIXME: Move to their own namespace
|
|
void modrinthEnsureMetadata(SequentialTask&, QByteArray&);
|
|
void flameEnsureMetadata(SequentialTask&, QByteArray&);
|
|
|
|
// Helpers
|
|
void emitReady();
|
|
void emitFail();
|
|
|
|
signals:
|
|
void metadataReady();
|
|
void metadataFailed();
|
|
|
|
private:
|
|
Mod& m_mod;
|
|
QDir& m_index_dir;
|
|
ModPlatform::Provider m_provider;
|
|
bool m_try_all;
|
|
|
|
MultipleOptionsTask* m_task_handler = nullptr;
|
|
};
|