Initial FTB support. Allows "tracking" of FTB instances.
This commit is contained in:
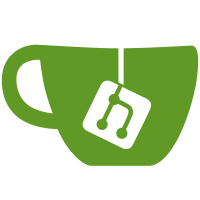
committed by
Petr Mrázek
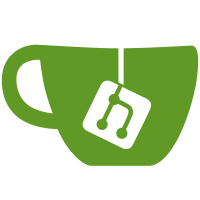
parent
34a3fedf7b
commit
82c87aa06f
77
logic/tasks/SequentialTask.cpp
Normal file
77
logic/tasks/SequentialTask.cpp
Normal file
@ -0,0 +1,77 @@
|
||||
#include "SequentialTask.h"
|
||||
|
||||
SequentialTask::SequentialTask(QObject *parent) :
|
||||
Task(parent), m_currentIndex(-1)
|
||||
{
|
||||
|
||||
}
|
||||
|
||||
QString SequentialTask::getStatus() const
|
||||
{
|
||||
if (m_queue.isEmpty() || m_currentIndex >= m_queue.size())
|
||||
{
|
||||
return QString();
|
||||
}
|
||||
return m_queue.at(m_currentIndex)->getStatus();
|
||||
}
|
||||
|
||||
void SequentialTask::getProgress(qint64 ¤t, qint64 &total)
|
||||
{
|
||||
current = 0;
|
||||
total = 0;
|
||||
for (int i = 0; i < m_queue.size(); ++i)
|
||||
{
|
||||
qint64 subCurrent, subTotal;
|
||||
m_queue.at(i)->getProgress(subCurrent, subTotal);
|
||||
current += subCurrent;
|
||||
total += subTotal;
|
||||
}
|
||||
}
|
||||
|
||||
void SequentialTask::addTask(std::shared_ptr<Task> task)
|
||||
{
|
||||
m_queue.append(task);
|
||||
}
|
||||
|
||||
void SequentialTask::executeTask()
|
||||
{
|
||||
m_currentIndex = -1;
|
||||
startNext();
|
||||
}
|
||||
|
||||
void SequentialTask::startNext()
|
||||
{
|
||||
if (m_currentIndex != -1)
|
||||
{
|
||||
std::shared_ptr<Task> previous = m_queue[m_currentIndex];
|
||||
disconnect(previous.get(), 0, this, 0);
|
||||
}
|
||||
m_currentIndex++;
|
||||
if (m_queue.isEmpty() || m_currentIndex >= m_queue.size())
|
||||
{
|
||||
emitSucceeded();
|
||||
return;
|
||||
}
|
||||
std::shared_ptr<Task> next = m_queue[m_currentIndex];
|
||||
connect(next.get(), SIGNAL(failed(QString)), this, SLOT(subTaskFailed(QString)));
|
||||
connect(next.get(), SIGNAL(status(QString)), this, SLOT(subTaskStatus(QString)));
|
||||
connect(next.get(), SIGNAL(progress(qint64,qint64)), this, SLOT(subTaskProgress()));
|
||||
connect(next.get(), SIGNAL(succeeded()), this, SLOT(startNext()));
|
||||
next->start();
|
||||
emit status(getStatus());
|
||||
}
|
||||
|
||||
void SequentialTask::subTaskFailed(const QString &msg)
|
||||
{
|
||||
emitFailed(msg);
|
||||
}
|
||||
void SequentialTask::subTaskStatus(const QString &msg)
|
||||
{
|
||||
setStatus(msg);
|
||||
}
|
||||
void SequentialTask::subTaskProgress()
|
||||
{
|
||||
qint64 current, total;
|
||||
getProgress(current, total);
|
||||
setProgress(100 * current / total);
|
||||
}
|
32
logic/tasks/SequentialTask.h
Normal file
32
logic/tasks/SequentialTask.h
Normal file
@ -0,0 +1,32 @@
|
||||
#pragma once
|
||||
|
||||
#include "Task.h"
|
||||
|
||||
#include <QQueue>
|
||||
#include <memory>
|
||||
|
||||
class SequentialTask : public Task
|
||||
{
|
||||
Q_OBJECT
|
||||
public:
|
||||
explicit SequentialTask(QObject *parent = 0);
|
||||
|
||||
virtual QString getStatus() const;
|
||||
virtual void getProgress(qint64 ¤t, qint64 &total);
|
||||
|
||||
void addTask(std::shared_ptr<Task> task);
|
||||
|
||||
protected:
|
||||
void executeTask();
|
||||
|
||||
private
|
||||
slots:
|
||||
void startNext();
|
||||
void subTaskFailed(const QString &msg);
|
||||
void subTaskStatus(const QString &msg);
|
||||
void subTaskProgress();
|
||||
|
||||
private:
|
||||
QQueue<std::shared_ptr<Task> > m_queue;
|
||||
int m_currentIndex;
|
||||
};
|
Reference in New Issue
Block a user